Show code cell source
# -*- coding: utf-8 -*-
# This is a report using the data from IQAASL.
# IQAASL was a project funded by the Swiss Confederation
# It produces a summary of litter survey results for a defined region.
# These charts serve as the models for the development of plagespropres.ch
# The data is gathered by volunteers.
# Please remember all copyrights apply, please give credit when applicable
# The repo is maintained by the community effective January 01, 2022
# There is ample opportunity to contribute, learn and teach
# contact dev@hammerdirt.ch
# Dies ist ein Bericht, der die Daten von IQAASL verwendet.
# IQAASL war ein von der Schweizerischen Eidgenossenschaft finanziertes Projekt.
# Es erstellt eine Zusammenfassung der Ergebnisse der Littering-Umfrage für eine bestimmte Region.
# Diese Grafiken dienten als Vorlage für die Entwicklung von plagespropres.ch.
# Die Daten werden von Freiwilligen gesammelt.
# Bitte denken Sie daran, dass alle Copyrights gelten, bitte geben Sie den Namen an, wenn zutreffend.
# Das Repo wird ab dem 01. Januar 2022 von der Community gepflegt.
# Es gibt reichlich Gelegenheit, etwas beizutragen, zu lernen und zu lehren.
# Kontakt dev@hammerdirt.ch
# Il s'agit d'un rapport utilisant les données de IQAASL.
# IQAASL était un projet financé par la Confédération suisse.
# Il produit un résumé des résultats de l'enquête sur les déchets sauvages pour une région définie.
# Ces tableaux ont servi de modèles pour le développement de plagespropres.ch
# Les données sont recueillies par des bénévoles.
# N'oubliez pas que tous les droits d'auteur s'appliquent, veuillez indiquer le crédit lorsque cela est possible.
# Le dépôt est maintenu par la communauté à partir du 1er janvier 2022.
# Il y a de nombreuses possibilités de contribuer, d'apprendre et d'enseigner.
# contact dev@hammerdirt.ch
# sys, file and nav packages:
import datetime as dt
from datetime import date, datetime, time
from babel.dates import format_date, format_datetime, format_time, get_month_names
import locale
# math packages:
import pandas as pd
import numpy as np
from math import pi
import scipy.stats as stats
# charting:
import matplotlib as mpl
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
from matplotlib import ticker
from matplotlib.ticker import MultipleLocator
import seaborn as sns
from matplotlib import colors as mplcolors
# build report
import reportlab
from reportlab.platypus.flowables import Flowable
from reportlab.platypus import SimpleDocTemplate, Paragraph, Spacer, Image, PageBreak, KeepTogether
from reportlab.lib.pagesizes import A4
from reportlab.rl_config import defaultPageSize
from reportlab.lib.units import inch, cm
from reportlab.lib.styles import getSampleStyleSheet, ParagraphStyle
from reportlab.lib.colors import HexColor
from reportlab.platypus import Table, TableStyle
from reportlab.lib import colors
# the module that has all the methods for handling the data
import resources.featuredata as featuredata
from resources.featuredata import makeAList
# home brew utitilties
import resources.chart_kwargs as ck
import resources.sr_ut as sut
# images and display
from IPython.display import Markdown as md
from PIL import Image as PILImage
from myst_nb import glue
# chart style
sns.set_style("whitegrid")
# border and row shading for tables
a_color = "saddlebrown"
table_row = "saddlebrown"
# a place to save figures and a
# method to choose formats
save_fig_prefix = "resources/output/"
# the arguments for formatting the image
save_figure_kwargs = {
"fname": None,
"dpi": 300.0,
"format": "jpeg",
"bbox_inches": None,
"pad_inches": 0,
"bbox_inches": 'tight',
"facecolor": 'auto',
"edgecolor": 'auto',
"backend": None,
}
## !! Begin Note book variables !!
# There are two language variants: german and english
# change both: date_lang and language
date_lang = 'de_DE.utf8'
locale.setlocale(locale.LC_ALL, date_lang)
# the date format of the survey data is defined in the module
date_format = featuredata.date_format
# the language setting use lower case: en or de
# changing the language may require changing the unit label
language = "de"
unit_label = "p/100 m"
# the standard date format is "%Y-%m-%d" if your date column is
# not in this format it will not work.
# these dates cover the duration of the IQAASL project
start_date = "2020-03-01"
end_date ="2021-05-31"
start_end = [start_date, end_date]
# the fail rate used to calculate the most common codes is
# 50% it can be changed:
fail_rate = 50
# Changing these variables produces different reports
# Call the map image for the area of interest
bassin_map = "resources/maps/all_survey_areas_summary.jpeg"
# the label for the aggregation of all data in the region
top = "Alle Erhebungsgebiete"
# define the feature level and components
# the feature of interest is the all (all) at the river basin (river_bassin) level.
# the label for charting is called 'name'
this_feature = {'slug':'all', 'name':"Alle Erhebungsgebiete", 'level':'all'}
# these are the smallest aggregated components
# choices are water_name_slug=lake or river, city or location at the scale of a river bassin
# water body or lake maybe the most appropriate
this_level = 'river_bassin'
# the doctitle is the unique name for the url of this document
doc_title = "lakes_rivers"
# # identify the lakes of interest for the survey area
# lakes_of_interest = ["neuenburgersee", "thunersee", "bielersee", "brienzersee"]
# !! End note book variables !!
## data
# Survey location details (GPS, city, land use)
dfBeaches = pd.read_csv("resources/beaches_with_land_use_rates.csv")
# set the index of the beach data to location slug
dfBeaches.set_index("slug", inplace=True)
# Survey dimensions and weights
dfDims = pd.read_csv("resources/corrected_dims.csv")
# code definitions
dxCodes = pd.read_csv("resources/codes_with_group_names_2015.csv")
dxCodes.set_index("code", inplace=True)
# columns that need to be renamed. Setting the language will automatically
# change column names, code descriptions and chart annotations
columns={"% to agg":"% agg", "% to recreation": "% recreation", "% to woods":"% woods", "% to buildings":"% buildings", "p/100m":"p/100 m"}
# !key word arguments to construct feature data
# !Note the water type allows the selection of river or lakes
# if None then the data is aggregated together. This selection
# is only valid for survey-area reports or other aggregated data
# that may have survey results from both lakes and rivers.
fd_kwargs ={
"filename": "resources/checked_sdata_eos_2020_21.csv",
"feature_name": this_feature['slug'],
"feature_level": this_feature['level'],
"these_features": this_feature['slug'],
"component": this_level,
"columns": columns,
"language": 'de',
"unit_label": unit_label,
"fail_rate": fail_rate,
"code_data":dxCodes,
"date_range": start_end,
"water_type": None,
}
fdx = featuredata.Components(**fd_kwargs)
# call the reports and languages
fdx.adjustForLanguage()
fdx.makeFeatureData()
fdx.locationSampleTotals()
fdx.makeDailyTotalSummary()
fdx.materialSummary()
fdx.mostCommon()
fdx.codeGroupSummary()
# !this is the feature data!
fd = fdx.feature_data
# !keyword args to build period data
# the period data is all the data that was collected
# during the same period from all the other locations
# not included in the feature data. For a survey area
# or river bassin these_features = feature_parent and
# feature_level = parent_level
period_kwargs = {
"period_data": fdx.period_data,
"these_features": this_feature['slug'],
"feature_level":this_feature['level'],
"feature_parent":this_feature['slug'],
"parent_level": this_feature['level'],
"period_name": top,
"unit_label": unit_label,
"most_common": fdx.most_common.index
}
period_data = featuredata.PeriodResults(**period_kwargs)
# the rivers are considered separately
# select only the results from rivers
fd_kwargs.update({"water_type":"r"})
fdr = featuredata.Components(**fd_kwargs)
fdr.makeFeatureData()
fdr.adjustForLanguage()
fdr.makeFeatureData()
fdr.locationSampleTotals()
fdr.makeDailyTotalSummary()
fdr.materialSummary()
fdr.mostCommon()
# collects the summarized values for the feature data
# use this to generate the summary data for the survey area
# and the section for the rivers
admin_kwargs = {
"data":fd,
"dims_data":dfDims,
"label": this_feature["name"],
"feature_component": this_level,
"date_range":start_end,
**{"dfBeaches":dfBeaches}
}
admin_details = featuredata.AdministrativeSummary(**admin_kwargs)
admin_summary = admin_details.summaryObject()
# update the admin kwargs with river data to make the river summary
admin_kwargs.update({"data":fdr.feature_data})
admin_r_details = featuredata.AdministrativeSummary(**admin_kwargs)
admin_r_summary = admin_r_details.summaryObject()
# this defines the css rules for the note-book table displays
header_row = {'selector': 'th:nth-child(1)', 'props': f'background-color: #FFF;text-align:right;'}
even_rows = {"selector": 'tr:nth-child(even)', 'props': f'background-color: rgba(139, 69, 19, 0.08);'}
odd_rows = {'selector': 'tr:nth-child(odd)', 'props': 'background: #FFF;'}
table_font = {'selector': 'tr', 'props': 'font-size: 12px;'}
table_data = {'selector': 'td', 'props': 'padding:6px;'}
table_css_styles = [even_rows, odd_rows, table_font, header_row, table_data]
# this defines the css rules for the note-book table displays
header_row = {'selector': 'th:nth-child(1)', 'props': f'background-color: #FFF;text-align:right;'}
table_font = {'selector': 'tr', 'props': 'font-size: 12px;'}
table_data = {'selector': 'td', 'props': 'padding:6px;'}
heat_map_css_styles = [table_font, header_row, table_data]
# pdf download is an option
# the .pdf output is generated in parallel
# this is the same as if it were on the backend where we would
# have a specific api endpoint for .pdf requests.
# reportlab is used to produce the document
# the components of the document are captured at run time
# the pdf link gives the name and location of the future doc
pdf_link = f'resources/pdfs/{this_feature["slug"]}_sa.pdf'
# the components are stored in an array and collected as the script runs
pdfcomponents = []
# pdf title and map
pdf_title = Paragraph(this_feature["name"], featuredata.title_style)
map_image = Image(bassin_map, width=cm*19, height=20*cm, kind="proportional", hAlign= "CENTER")
map_caption = featuredata.defaultMapCaption(language="de")
f1cap = Paragraph(map_caption, featuredata.caption_style),
glue(f'{this_feature["slug"]}_city_map_caption', map_caption, display=False)
glue("blank_caption", " ", display=False)
def convertPixelToCm(file_name: str = None):
im = PILImage.open(file_name)
width, height = im.size
dpi = im.info.get("dpi", (72, 72))
width_cm = width / dpi[0] * 2.54
height_cm = height / dpi[1] * 2.54
return width_cm, height_cm
o_w, o_h = convertPixelToCm(bassin_map)
f1cap = Paragraph(map_caption, style=featuredata.caption_style)
figure_kwargs = {
"image_file":bassin_map,
"caption": f1cap,
"original_width":o_w,
"original_height":o_h,
"desired_width": 16,
"caption_height":.75,
"hAlign": "CENTER",
}
f1 = featuredata.figureAndCaptionTable(**figure_kwargs)
pdfcomponents = featuredata.addToDoc([
pdf_title,
featuredata.small_space,
f1
], pdfcomponents)
1. Seen und Fliessgewässer#
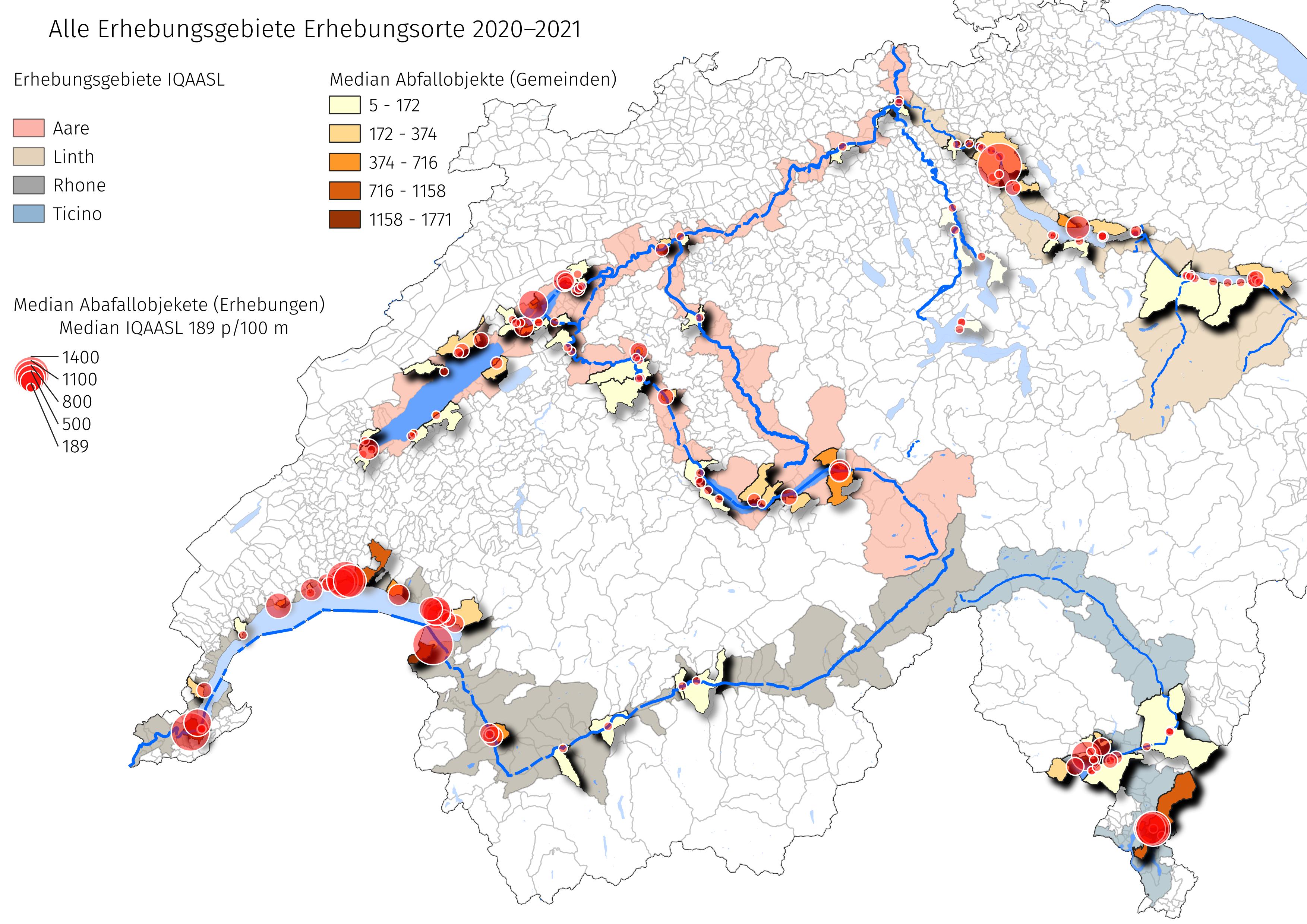
Abb. 1.1 #
Abbildung 1.1: Die Erhebungsorte sind für die Analyse nach Erhebungsgebiet gruppiert. Die Grösse der Markierung gibt einen Hinweis auf die Anzahl Abfallobjekte, die gefunden wurden.
Show code cell source
# the admin summary can be converted into a standard text
an_admin_summary = featuredata.makeAdminSummaryStateMent(start_date, end_date, this_feature["name"], admin_summary=admin_summary)
# collect component features and land marks
# this collects the components of the feature of interest (city, lake, river)
# a comma separated string of all the componenets and a heading for each component
# type is produced
feature_components = featuredata.collectComponentLandMarks(admin_details, language="de")
# markdown output
components_markdown = "".join([f'*{x[0]}*\n\n>{x[1]}\n\n' for x in feature_components])
new_components = [
featuredata.small_space,
Paragraph("Erhebungsorte", featuredata.section_title),
featuredata.smallest_space,
Paragraph(an_admin_summary , featuredata.p_style),
]
# add the admin summary to the pdf
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
# put that all together:
lake_string = F"""
{an_admin_summary}
{"".join(components_markdown)}
"""
md(lake_string)
Im Zeitraum von März 2020 bis Mai 2021 wurden im Rahmen von 386 Datenerhebungen insgesamt 54 744 Objekte entfernt und identifiziert. Die Ergebnisse des Alle Erhebungsgebiete umfassen 143 Orte, 77 Gemeinden und eine Gesamtbevölkerung von etwa 1 735 991 Einwohnenden.
Seen
Zürichsee, Lac Léman, Lago Maggiore, Thunersee, Bielersee, Lago di Lugano, Neuenburgersee, Walensee, Brienzersee, Vierwaldstättersee, Zugersee
Fliessgewässer
Aare, Nidau-Büren-Kanal, Ticino, Cassarate, Jona, Dorfbach, La Thièle, Rhône, Limmat, Linthkanal, Escherkanal, Reuss, Maggia, Schüss, Emme, Seez, Sihl
Gemeinden
Aarau, Allaman, Ascona, Beatenberg, Bellinzona, Bern, Biel/Bienne, Boudry, Bourg-en-Lavaux, Brienz (BE), Brissago, Brugg, Brügg, Burgdorf, Bönigen, Cheyres-Châbles, Cudrefin, Dietikon, Erlach, Estavayer, Freienbach, Gals, Gambarogno, Gebenstorf, Genève, Gland, Glarus Nord, Grandson, Hauterive (NE), Hünenberg, Kallnach, Köniz, Küsnacht (ZH), La Tour-de-Peilz, Lausanne, Lavey-Morcles, Le Landeron, Leuk, Ligerz, Locarno, Lugano, Luterbach, Lüscherz, Merenschwand, Minusio, Montreux, Neuchâtel, Nidau, Port, Préverenges, Quarten, Rapperswil-Jona, Richterswil, Riddes, Rubigen, Saint-Gingolph, Saint-Sulpice (VD), Salgesch, Schmerikon, Sion, Solothurn, Spiez, Stäfa, Thun, Tolochenaz, Unterengstringen, Unterseen, Versoix, Vevey, Vinelz, Walenstadt, Walperswil, Weesen, Weggis, Yverdon-les-Bains, Zug, Zürich
1.1. Landnutzungsprofil#
Die Erhebungsgebiete sind nach Flusseinzugsgebieten gruppiert. In diesem Bericht werden mehrere Einzugsgebiete zusammengefasst, um regionale Trends zu analysieren:
Aare: Emme, Aare
Linth: Reuss, Linth, Limmat
Rhône: Rhône
Tessin/Ceresio: Tessin, Luganersee, Lago Maggiore
Das Landnutzungsprofil zeigt, welche Nutzungen innerhalb eines Radius von 1500 m um jeden Erhebungsort dominieren. Flächen werden einer von den folgenden vier Kategorien zugewiesen:.
Fläche, die von Gebäuden eingenommen wird in %
Fläche, die dem Wald vorbehalten ist in %
Fläche, die für Aktivitäten im Freien genutzt wird in %
Fläche, die von der Landwirtschaft genutzt wird in %
Strassen (inkl. Wege) werden als Gesamtzahl der Strassenkilometer innerhalb eines Radius von 1500 m angegeben.
Es wird zudem angegeben, wie viele Flüsse innerhalb eines Radius von 1500 m um den Erhebungsort herum in das Gewässer münden.
Das Verhältnis der gefundenen Abfallobjekte unterscheidet sich je nach Landnutzungsprofil. Das Verhältnis gibt daher einen Hinweis auf die ökologischen und wirtschaftlichen Bedingungen um den Erhebungsort.
Weitere Informationen gibt es im Kapitel Landnutzungsprofil
Verteilung der Landnutzungsmerkmale
Show code cell source
# the land use data per location (for all locations) for a radius of 1 500 m is
# available woth the survey results. Using the period data gets the land use
# rates for all locations (not just the feature of interest)
land_use_kwargs = {
"data": period_data.period_data,
"index_column":"loc_date",
"these_features": this_feature['slug'],
"feature_level":this_level
}
# the landuse profile of the project
project_profile = featuredata.LandUseProfile(**land_use_kwargs).byIndexColumn()
# change the data in the land_use_kargs to get different rates
# update the kwargs for the feature data
land_use_kwargs.update({"data":fdx.feature_data})
# build the landuse profile of the feature
feature_profile = featuredata.LandUseProfile(**land_use_kwargs)
# this is the component features of the report
feature_landuse = feature_profile.featureOfInterest()
fig, axs = plt.subplots(2, 3, figsize=(9,8), sharey="row")
for i, n in enumerate(featuredata.default_land_use_columns):
r = i%2
c = i%3
ax=axs[r,c]
# the value of land use feature n
# for each element of the feature
for element in feature_landuse:
# the land use data for a feature
data = element[n].values
# the name of the element
element_name = element[feature_profile.feature_level].unique()
# proper name for chart
label = featuredata.river_basin_de[element_name[0]]
# cumulative distribution
xs, ys = featuredata.empiricalCDF(data)
# the plot of landuse n for this element
sns.lineplot(x=xs, y=ys, ax=ax, label=label, color=featuredata.bassin_pallette[element_name[0]])
# the value of the land use feature n for the project
testx, testy = featuredata.empiricalCDF(project_profile[n].values)
sns.lineplot(x=testx, y=testy, ax=ax, label=top, color="magenta")
# get the median landuse for the feature
the_median = np.median(data)
# plot the median and drop horizontal and vertical lines
ax.scatter([the_median], 0.5, color="red",s=40, linewidth=1, zorder=100, label="Median")
ax.vlines(x=the_median, ymin=0, ymax=0.5, color="red", linewidth=1)
ax.hlines(xmax=the_median, xmin=0, y=0.5, color="red", linewidth=1)
if i <= 3:
if c == 0:
ax.yaxis.set_major_locator(MultipleLocator(.1))
ax.xaxis.set_major_formatter(ticker.PercentFormatter(1.0, 0, "%"))
else:
pass
handles, labels = ax.get_legend_handles_labels()
ax.get_legend().remove()
ax.set_title(featuredata.luse_de[n], loc='left')
plt.tight_layout()
plt.subplots_adjust(top=.91, hspace=.5)
plt.suptitle("Landnutzung im Umkries von 1 500 m um den Erhebungsort", ha="center", y=1, fontsize=16)
fig.legend(handles, labels, bbox_to_anchor=(.5,.5), loc="upper center", ncol=6)
figure_caption = [
f"Die Erhebungen in den Gebieten Rhône und Linth wiesen mit {'47 %'} bzw. {'40 %'} im ",
f"Median den grössten Anteil an bebauter Fläche (Gebäude) und mit {'5 %'} bzw. {'8 %'} ",
"den geringsten Anteil an Wald auf. Im Erhebungsgebiet Aare war der Medianwert der ",
f"bebauten Fläche (Gebäude) mit {'16 %'} am niedrigsten und der Anteil der landwirtschaftlich ",
f"genutzten Fläche mit {'30 %'} am höchsten. Bei den Flächen, die den Aktivitäten im Freien ",
"zugeordnet werden, handelt es sich um Sportplätze, öffentliche Strände und andere öffentliche Versammlungsorte."
]
land_use_figure_caption = ''.join(figure_caption)
figure_name = "all_survey_area_landuse"
land_use_file_name = f"{save_fig_prefix}{figure_name}.jpeg"
save_figure_kwargs.update({"fname":land_use_file_name})
print(land_use_file_name)
plt.tight_layout()
plt.subplots_adjust(top=.91, hspace=.4)
plt.savefig(**save_figure_kwargs)
# capture the output
glue( f'{this_feature["slug"]}_survey_area_landuse', fig, display=False)
glue(f'{this_feature["slug"]}_land_use_caption', land_use_figure_caption, display=False)
plt.close()
resources/output/all_survey_area_landuse.jpeg
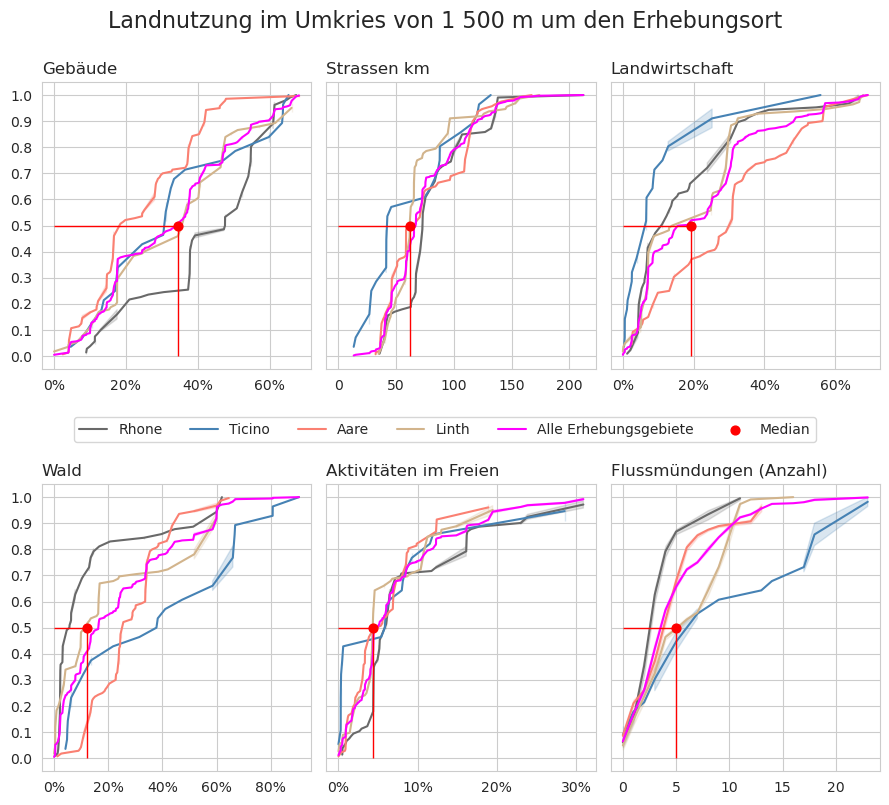
Abb. 1.2 #
Abbildung 1.2: Die Erhebungen in den Gebieten Rhône und Linth wiesen mit 47 % bzw. 40 % im Median den grössten Anteil an bebauter Fläche (Gebäude) und mit 5 % bzw. 8 % den geringsten Anteil an Wald auf. Im Erhebungsgebiet Aare war der Medianwert der bebauten Fläche (Gebäude) mit 16 % am niedrigsten und der Anteil der landwirtschaftlich genutzten Fläche mit 30 % am höchsten. Bei den Flächen, die den Aktivitäten im Freien zugeordnet werden, handelt es sich um Sportplätze, öffentliche Strände und andere öffentliche Versammlungsorte.
1.1.1. Gesamtergebnisse nach Erhebungsgebiet#
Show code cell source
# the dimensional data
dims_table = admin_details.dimensionalSummary()
# a method to update the place names from slug to proper name
name_map = featuredata.river_basin_de
# the order in which they are charted
name_order = list(name_map.keys())
# sort by quantity
dims_table.sort_values(by=["quantity"], ascending=False, inplace=True)
# apply language settings
dims_table.rename(columns=featuredata.dims_table_columns_de, inplace=True)
# convert to kilos
dims_table["Plastik (Kg)"] = dims_table["Plastik (Kg)"]/1000
# save a copy of the dims_table for working
# formatting to pdf will turn the numerics to strings
# which eliminates any further calclations
dims_df = dims_table.copy()
# these columns need formatting for locale
thousands_separated = ["Fläche (m2)", "Länge (m)", "Erhebungen", "Objekte (St.)"]
replace_decimal = ["Plastik (Kg)", "Gesamtgewicht (Kg)"]
# format the dimensional summary for .pdf and add to components
dims_table[thousands_separated] = dims_table[thousands_separated].applymap(lambda x: featuredata.thousandsSeparator(int(x), "de"))
dims_table[replace_decimal] = dims_table[replace_decimal].applymap(lambda x: featuredata.replaceDecimal(str(round(x,2))))
# subsection title
subsection_title = Paragraph("Gesamtergebnisse nach Erhebungsgebiet", featuredata.subsection_title)
# a caption for the figure
caption_kwargs = {
"name": "caption_style",
"fontSize": 9,
"fontName": "Times-Italic",
"leftIndent":6
}
new_caption_style = ParagraphStyle(**caption_kwargs)
dims_table_caption = [
"Summen der Ergebnisse für alle Erhebungsgebiete. Im Gebiet Aare ist die ",
"Anzahl Erhebungen am grössten. Gleichzeitig ist dort die Anzahl gesammelter ",
"Objekte pro Fläche am geringsten."
]
# pdf table out
dims_table_caption = ''.join(dims_table_caption)
col_widths = [3.5*cm, 3*cm, *[2.2*cm]*(len(dims_table.columns)-1)]
dims_pdf_table = featuredata.aSingleStyledTable(dims_table, colWidths=col_widths, style=featuredata.default_table_style)
dims_pdf_table_caption = Paragraph(dims_table_caption, new_caption_style)
dims_pdf_table_and_caption = featuredata.tableAndCaption(dims_pdf_table, dims_pdf_table_caption,col_widths)
new_components = [
featuredata.large_space,
subsection_title,
featuredata.small_space,
dims_pdf_table_and_caption
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
# end pdf table
# this formats the table through the data frame
dims_df["Plastik (Kg)"] = dims_df["Plastik (Kg)"].round(2)
dims_df["Gesamtgewicht (Kg)"] = dims_df["Gesamtgewicht (Kg)"].round(2)
dims_df[thousands_separated] = dims_df[thousands_separated].astype("int")
# set the index name to None so it doesn't show in the columns
dims_df.index.name = None
dims_df.columns.name = None
# this applies formatting to the specifc column based on
# the language.
dims_table_formatter = {
"Plastik (Kg)": lambda x: featuredata.replaceDecimal(x, "de"),
"Gesamtgewicht (Kg)": lambda x: featuredata.replaceDecimal(x, "de"),
"Fläche (m2)": lambda x: featuredata.thousandsSeparator(int(x), "de"),
"Länge (m)": lambda x: featuredata.thousandsSeparator(int(x), "de"),
"Erhebungen": lambda x: featuredata.thousandsSeparator(int(x), "de"),
"Objekte (St.)": lambda x: featuredata.thousandsSeparator(int(x), "de")
}
# use the caption from the .pdf for the online figure
glue(f'{this_feature["slug"]}_dims_table_caption',dims_table_caption, display=False)
# apply formatting and styles to dataframe
q = dims_df.style.format(formatter=dims_table_formatter).set_table_styles(table_css_styles)
# capture the figure before display and give it a reference number and caption
figure_name=f'{this_feature["slug"]}_dims_table'
glue(figure_name, q, display=False)
Gesamtgewicht (Kg) | Plastik (Kg) | Fläche (m2) | Länge (m) | Erhebungen | Objekte (St.) | |
---|---|---|---|---|---|---|
Alle Erhebungsgebiete | 305,51 | 94,21 | 96 616 | 19 722 | 386 | 54 744 |
rhone | 151,31 | 45,97 | 25 986 | 4 911 | 106 | 28 454 |
aare | 71,98 | 31,45 | 37 017 | 7 971 | 140 | 13 847 |
linth | 35,96 | 12,77 | 25 637 | 5 323 | 112 | 9 412 |
ticino | 46,26 | 4,03 | 7 974 | 1 517 | 28 | 3 031 |
Abb. 1.3 #
Abbildung 1.3: Summen der Ergebnisse für alle Erhebungsgebiete. Im Gebiet Aare ist die Anzahl Erhebungen am grössten. Gleichzeitig ist dort die Anzahl gesammelter Objekte pro Fläche am geringsten.
1.2. Erhebungsergebnisse für alle Objekte#
Verteilung der Erhebungsergebnisse. Die Werte werden als Anzahl der identifizierten Abfallobjekte pro 100 Meter (p/100 m) dargestellt.
Show code cell source
# the sample totals of the parent feautre
dx = period_data.parentSampleTotals(parent=False)
# get the monthly or quarterly results for the feature
rsmp = fdx.sample_totals.set_index("date")
resample_plot, rate = featuredata.quarterlyOrMonthlyValues(rsmp, this_feature["name"], vals=unit_label)
fig, axs = plt.subplots(1,2, figsize=(11,5))
ax = axs[0]
# axis notations
months = mdates.MonthLocator(interval=1)
months_fmt = mdates.DateFormatter("%b")
days = mdates.DayLocator(interval=7)
# adjust the pallette
bassin_pallette = {k:v for k, v in featuredata.bassin_pallette.items() if k in fdx.sample_totals.river_bassin.unique()}
name_order = bassin_pallette.keys()
# feature surveys
sns.scatterplot(data=fdx.sample_totals, x="date", y=unit_label, hue='river_bassin', hue_order=name_order, palette=bassin_pallette, s=34, ec="white", ax=ax)
# monthly or quaterly plot
sns.lineplot(data=resample_plot, x=resample_plot.index, y=resample_plot, label=F"{this_feature['name']}: monatlicher Medianwert", color="magenta", ax=ax)
# label the yaxis
ax.set_ylabel(unit_label, **{'labelpad':10, 'fontsize':12})
# label the xaxis
ax.set_xlabel("")
# format ticks and locations
ax.xaxis.set_minor_locator(days)
ax.xaxis.set_major_formatter(months_fmt)
ax.set_ylim(-50, 1500)
# the legend labels need to be changed to proper names
h, l = ax.get_legend_handles_labels()
new_labels = [*[name_map[x] for x in l[:-1]], l[-1]]
# apply new labels and legend
ax.legend(h, new_labels, bbox_to_anchor=(0, .98), loc="upper left", ncol=1, framealpha=.6)
# the cumlative distributions:
axtwo = axs[1]
# for each survey area:
for basin in name_map.keys():
# filter the data for the element
mask = fdx.sample_totals.river_bassin == basin
ecdf_data = fdx.sample_totals[mask]
# make the ECDF and make the plot
feature_ecd = featuredata.ecdfOfAColumn(ecdf_data, unit_label)
sns.lineplot(x=feature_ecd["x"], y=feature_ecd["y"], color=featuredata.bassin_pallette[basin], ax=axtwo, label=name_map[basin])
# the ecdf of the parent element
other_features = featuredata.ecdfOfAColumn(dx, unit_label)
sns.lineplot(x=other_features["x"], y=other_features["y"], color="magenta", label=top, linewidth=1, ax=axtwo)
# the axist labels
axtwo.set_xlabel(unit_label, **ck.xlab_k14)
axtwo.set_ylabel("Verhältnis der Erhebungen", **ck.xlab_k14)
# set the limit of the xaxis, other wise it can go on forever
axtwo.set_xlim(0, 3000)
# set and locate the ticks on the x and y axis
axtwo.xaxis.set_major_locator(MultipleLocator(500))
axtwo.xaxis.set_minor_locator(MultipleLocator(100))
axtwo.yaxis.set_major_locator(MultipleLocator(.1))
# format the minor gridlines
axtwo.grid(which="minor", visible=True, axis="x", linestyle="--", linewidth=1)
axtwo.legend(bbox_to_anchor=(.4,.5), loc="upper left")
plt.tight_layout()
figure_name = f"{this_feature['slug']}_sample_totals"
sample_totals_file_name = f'{save_fig_prefix}{figure_name}.jpeg'
save_figure_kwargs.update({"fname":sample_totals_file_name})
plt.savefig(**save_figure_kwargs)
# figure caption
sample_total_notes = [
"Links: Alle Erhebungen zwischen März 2020 bis Mai 2021, gruppiert nach ",
f"Erhebungsgebiet und aggregiert zum monatlichen Median. Werte über {'1 778 '}{unit_label} ",
"sind nicht dargestellt. Rechts: Die empirische Verteilungsfunktion der Gesamtwerte ",
"der Erhebungen."
]
sample_total_notes = ''.join(sample_total_notes)
glue(f'{this_feature["slug"]}_sample_total_notes', sample_total_notes, display=False)
glue(f'{this_feature["slug"]}_sample_totals', fig, display=False)
plt.close()
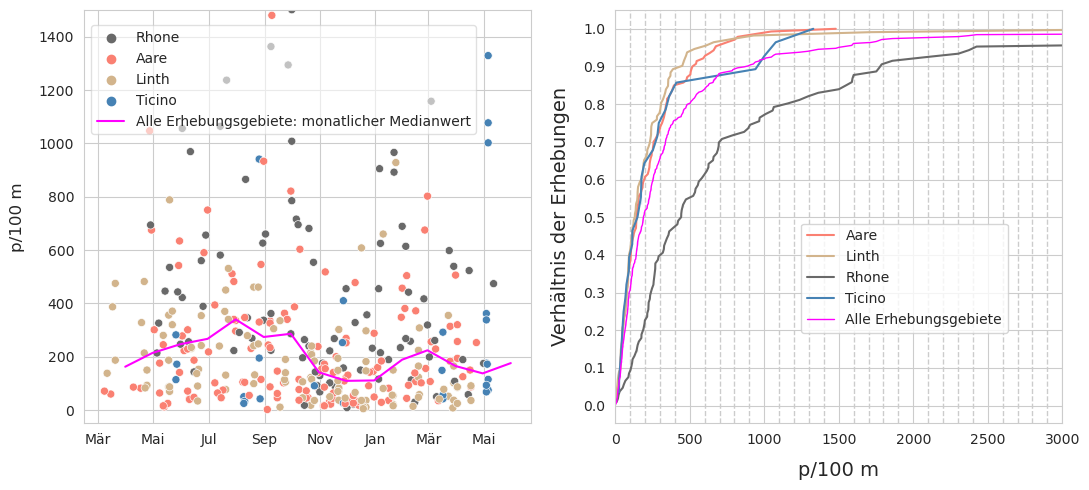
Abb. 1.4 #
Abbildung 1.4: Links: Alle Erhebungen zwischen März 2020 bis Mai 2021, gruppiert nach Erhebungsgebiet und aggregiert zum monatlichen Median. Werte über 1 778 p/100 m sind nicht dargestellt. Rechts: Die empirische Verteilungsfunktion der Gesamtwerte der Erhebungen.
Abfall nach Materialarten im Überblick
Show code cell source
# the summary of the sample totals
csx = fdx.sample_summary.copy()
# format for the current language
combined_summary =[(x, featuredata.thousandsSeparator(int(csx[x]), language)) for x in csx.index]
# the materials table
fd_mat_totals = fdx.material_summary.copy()
fd_mat_totals = featuredata.fmtPctOfTotal(fd_mat_totals, around=0)
# applly new column names for printing
cols_to_use = {"material":"Material","quantity":"Objekte (St.)", "% of total":"Anteil"}
fd_mat_t = fd_mat_totals[cols_to_use.keys()].values
fd_mat_t = [(x[0], featuredata.thousandsSeparator(int(x[1]), language), x[2]) for x in fd_mat_t]
# make tables
fig, axs = plt.subplots(1,2)
# names for the table columns
a_col = [this_feature["name"], "Total"]
axone = axs[0]
sut.hide_spines_ticks_grids(axone)
table_two = sut.make_a_table(axone, combined_summary, colLabels=a_col, colWidths=[.75,.25], bbox=[0,0,1,1], **{"loc":"lower center"})
table_two.get_celld()[(0,0)].get_text().set_text(" ")
table_two.set_fontsize(12)
# material table
axtwo = axs[1]
axtwo.set_xlabel(" ")
sut.hide_spines_ticks_grids(axtwo)
table_three = sut.make_a_table(axtwo, fd_mat_t, colLabels=list(cols_to_use.values()), colWidths=[.4, .4,.2], bbox=[0,0,1,1], **{"loc":"lower center"})
table_three.get_celld()[(0,0)].get_text().set_text(" ")
table_three.set_fontsize(12)
plt.tight_layout()
plt.subplots_adjust(wspace=0.05)
# figure caption
summary_of_survey_totals = [
"Links: Zusammenfassung der Resultate für alle Erhebungsgebiete. ",
"Rechts: Materialarten in Stückzahlen und als Prozentsatz an der ",
"Gesamtmenge (Stückzahl) für alle Erhebungsgebiete."
]
summary_of_survey_totals = ''.join(summary_of_survey_totals)
glue(f'{this_feature["slug"]}_sample_summaries_caption', summary_of_survey_totals, display=False)
figure_name = f'{this_feature["slug"]}_sample_summaries'
sample_summaries_file_name = f'{save_fig_prefix}{figure_name}.jpeg'
save_figure_kwargs.update({"fname":sample_summaries_file_name})
plt.savefig(**save_figure_kwargs)
glue(f'{this_feature["slug"]}_survey_area_sample_material_tables', fig, display=False)
plt.close()
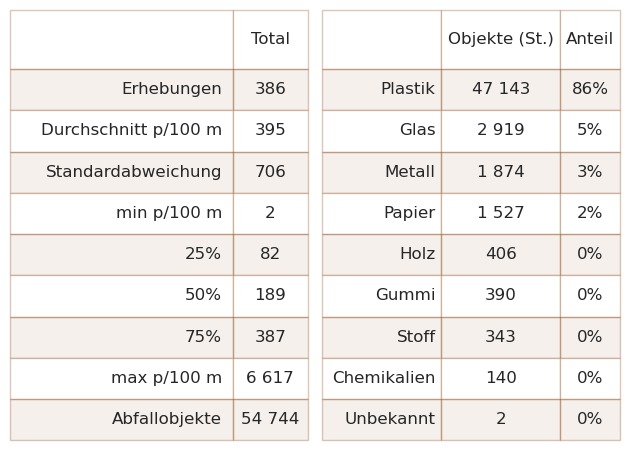
Abb. 1.5 #
Abbildung 1.5: Links: Zusammenfassung der Resultate für alle Erhebungsgebiete. Rechts: Materialarten in Stückzahlen und als Prozentsatz an der Gesamtmenge (Stückzahl) für alle Erhebungsgebiete.
1.3. Die am häufigsten gefundenen Gegenstände#
Die am häufigsten gefundenen Objekte sind die zehn mengenmässig am meisten vorkommenden Objekte und/oder Objekte, die in mindestens 50 % aller Datenerhebungen identifiziert wurden (Häufigkeitsrate).
Show code cell source
# add new section to pdf
mc_section_title = Paragraph("Die am häufigsten gefundenen Gegenstände", featuredata.section_title)
para_g = [
"Die am häufigsten gefundenen Objekte sind die zehn mengenmässig am meisten ",
f"vorkommenden Objekte und/oder Objekte, die in mindestens 50 % aller ",
"Datenerhebungen identifiziert wurden (Häufigkeitsrate)"
]
para_g = ''.join(para_g)
mc_section_para = Paragraph(para_g, featuredata.p_style)
# the most common objects results
most_common_display = fdx.most_common
# language appropriate columns
cols_to_use = featuredata.most_common_objects_table_de
cols_to_use.update({unit_label:unit_label})
# data for display
most_common_display.rename(columns=cols_to_use, inplace=True)
most_common_display = most_common_display[cols_to_use.values()].copy()
most_common_display = most_common_display.set_index("Objekte", drop=True)
# .pdf output
data = most_common_display.copy()
data["Anteil"] = data["Anteil"].map(lambda x: f"{int(x)}%")
data['Objekte (St.)'] = data['Objekte (St.)'].map(lambda x:featuredata.thousandsSeparator(x, language))
data['Häufigkeitsrate'] = data['Häufigkeitsrate'].map(lambda x: f"{x}%")
data[unit_label] = data[unit_label].map(lambda x: featuredata.replaceDecimal(round(x,1)))
dxi = data.copy()
# make caption
# get percent of total to make the caption string
mc_caption_string = [
"Die häufigsten Objekte für alle Erhebungsgebiete. Die Häufigkeitsrate gibt an, wie oft ein Objekt "
"in den Erhebungen im Verhältnis zu allen Erhebungen identifiziert wurde: Zigarettenfilter ",
f"beispielsweise wurden in {'87'} {'%'} der Erhebungen gefunden. Zusammengenommen machen die häufigsten ",
f"Objekte {'68'} {'%'} aller gefundenen Objekte aus."
]
mc_caption_string = "".join(mc_caption_string)
col_widths = [4.5*cm, 2.2*cm, 2*cm, 2.8*cm, 2*cm]
mc_table = featuredata.aSingleStyledTable(data, colWidths=col_widths)
mc_table_caption = Paragraph(mc_caption_string, new_caption_style)
t_and_c = featuredata.tableAndCaption(mc_table, mc_table_caption,col_widths)
new_components = [
KeepTogether([
mc_section_title,
featuredata.small_space,
mc_section_para
]),
featuredata.small_space,
t_and_c
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
most_common_display.index.name = None
most_common_display.columns.name = None
# set pandas display
aformatter = {
"Anteil":lambda x: f"{int(x)}%",
f"{unit_label}": lambda x: featuredata.replaceDecimal(x, "de"),
"Häufigkeitsrate": lambda x: f"{int(x)}%",
"Objekte (St.)": lambda x: featuredata.thousandsSeparator(int(x), "de")
}
mcd = most_common_display.style.format(aformatter).set_table_styles(table_css_styles)
glue(f'{this_feature["slug"]}_most_common_caption', mc_caption_string, display=False)
glue(f'{this_feature["slug"]}_most_common_tables', mcd, display=False)
Objekte (St.) | Anteil | Häufigkeitsrate | p/100 m | |
---|---|---|---|---|
Zigarettenfilter | 8 485 | 15% | 87% | 20,0 |
Fragmentierte Kunststoffe | 7 400 | 13% | 86% | 18,0 |
Expandiertes Polystyrol | 5 563 | 10% | 68% | 5,0 |
Snack-Verpackungen | 3 325 | 6% | 85% | 9,0 |
Industriefolie (Kunststoff) | 2 534 | 4% | 69% | 5,0 |
Getränkeflaschen aus Glas, Glasfragmente | 2 136 | 3% | 65% | 3,0 |
Industriepellets (Nurdles) | 1 968 | 3% | 30% | 0,0 |
Schaumstoffverpackungen/Isolierung | 1 702 | 3% | 53% | 1,0 |
Wattestäbchen/Tupfer | 1 406 | 2% | 50% | 1,0 |
Styropor < 5mm | 1 209 | 2% | 25% | 0,0 |
Kunststoff-Bauabfälle | 992 | 1% | 52% | 1,0 |
Kronkorken, Lasche von Dose/Ausfreisslachen | 700 | 1% | 52% | 1,0 |
Abb. 1.6 #
Abbildung 1.6: Die häufigsten Objekte für alle Erhebungsgebiete. Die Häufigkeitsrate gibt an, wie oft ein Objekt in den Erhebungen im Verhältnis zu allen Erhebungen identifiziert wurde: Zigarettenfilter beispielsweise wurden in 87 % der Erhebungen gefunden. Zusammengenommen machen die häufigsten Objekte 68 % aller gefundenen Objekte aus.
Häufigste Objekte im Median p/100 m nach Erhebungsgebiet
Show code cell source
mc_heat_map_caption = [
f'Der Median {unit_label} der häufigsten Objekte für alle Erhebungsgebiete. Die Werte ',
'für die jeweiligen Erhebungsgebiete unterscheiden sich deutlich.'
]
mc_heat_map_caption = ''.join(mc_heat_map_caption)
# calling componentsMostCommon gets the results for the most common codes
# at the component level
components = fdx.componentMostCommonPcsM()
# map to proper names for features
feature_names = featuredata.river_basin_de
# pivot that and quash the hierarchal column index that is created when the table is pivoted
mc_comp = components[["item", unit_label, this_level]].pivot(columns=this_level, index="item")
mc_comp.columns = mc_comp.columns.get_level_values(1)
# insert the proper columns names for display
proper_column_names = {x : feature_names[x] for x in mc_comp.columns}
mc_comp.rename(columns = proper_column_names, inplace=True)
# the aggregated total of the feature is taken from the most common objects table
mc_feature = fdx.most_common[unit_label]
mc_feature = featuredata.changeSeriesIndexLabels(mc_feature, {x:fdx.dMap.loc[x] for x in mc_feature.index})
# the aggregated totals of all the period data
mc_period = period_data.parentMostCommon(parent=False)
mc_period = featuredata.changeSeriesIndexLabels(mc_period, {x:fdx.dMap.loc[x] for x in mc_period.index})
# add the feature, bassin_label and period results to the components table
mc_comp["Alle Erhebungsgebiete"]= mc_feature
# mc_comp[top] = mc_period
# pdf out put
def splitTableWidth(data, caption_prefix: str = None, caption: str = None, gradient=False,
this_feature: str = None, vertical_header: bool = False, colWidths=[4*cm, *[None]*len(data.columns)]):
if len(data.columns) > 13:
tables = featuredata.aStyledTableExtended(data, gradient=gradient, caption_prefix=caption_prefix, vertical_header=vertical_header, colWidths=colWidths)
else:
mc_table = featuredata.aSingleStyledTable(data, colWidths=col_widths, vertical_header=True, gradient=gradient)
mc_table_caption = Paragraph(caption, new_caption_style)
table = featuredata.tableAndCaption(mc_table, mc_table_caption,col_widths)
return table
caption_prefix = f'Median {unit_label} der häufigsten Objekte am '
col_widths=[4.5*cm, *[1.2*cm]*(len(mc_comp.columns)-1)]
mc_heatmap_title = Paragraph(f"Häufigste Objekte im Median {unit_label} nach Erhebungsgebiet", featuredata.bold_block)
tables = splitTableWidth(mc_comp, gradient=True, caption_prefix=caption_prefix, caption=mc_heat_map_caption,
this_feature=this_feature["name"], vertical_header=True, colWidths=col_widths)
# identify the tables variable as either a list or a Flowable:
if isinstance(tables, list):
grouped_pdf_components = [*tables]
else:
grouped_pdf_components = [tables]
new_components = [
featuredata.small_space,
mc_heatmap_title,
featuredata.small_space,
*grouped_pdf_components
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
# notebook display style
aformatter = {x: featuredata.replaceDecimal for x in mc_comp.columns}
mcd = mc_comp.style.format(aformatter).set_table_styles(heat_map_css_styles)
mcd = mcd.background_gradient(axis=None, vmin=mc_comp.min().min(), vmax=mc_comp.max().max(), cmap="YlOrBr")
# remove the index name and column name labels
mcd.index.name = None
mcd.columns.name = None
# rotate the text on the header row
# the .applymap_index method in the
# df.styler module is used for this
mcd = mcd.applymap_index(featuredata.rotateText, axis=1)
# display markdown html
glue(f'{this_feature["slug"]}_mc_heat_map_caption', mc_heat_map_caption, display=False)
glue(f'{this_feature["slug"]}_most_common_heat_map', mcd, display=False)
Aare | Linth | Rhone | Ticino | Alle Erhebungsgebiete | |
---|---|---|---|---|---|
Getränkeflaschen aus Glas, Glasfragmente | 3,0 | 4,0 | 2,0 | 8,0 | 3,0 |
Expandiertes Polystyrol | 4,0 | 3,0 | 17,5 | 6,0 | 5,0 |
Fragmentierte Kunststoffe | 18,5 | 10,5 | 48,0 | 9,5 | 18,0 |
Industriefolie (Kunststoff) | 5,0 | 2,0 | 9,0 | 3,5 | 5,0 |
Industriepellets (Nurdles) | 0,0 | 0,0 | 0,0 | 0,0 | 0,0 |
Kronkorken, Lasche von Dose/Ausfreisslachen | 0,0 | 1,0 | 3,0 | 1,0 | 1,0 |
Kunststoff-Bauabfälle | 0,0 | 0,0 | 5,5 | 2,5 | 1,0 |
Schaumstoffverpackungen/Isolierung | 0,0 | 0,0 | 7,0 | 3,0 | 1,0 |
Snack-Verpackungen | 8,0 | 6,0 | 19,0 | 4,0 | 9,0 |
Styropor < 5mm | 0,0 | 0,0 | 0,0 | 0,0 | 0,0 |
Wattestäbchen/Tupfer | 0,0 | 0,0 | 10,5 | 0,0 | 1,0 |
Zigarettenfilter | 11,0 | 23,0 | 41,5 | 15,5 | 20,0 |
Abb. 1.7 #
Abbildung 1.7: Der Median p/100 m der häufigsten Objekte für alle Erhebungsgebiete. Die Werte für die jeweiligen Erhebungsgebiete unterscheiden sich deutlich.
Häufigste Objekte im Monatsdurchschnitt
Show code cell source
# collect the survey results of the most common objects
# and aggregate code with groupname for each sample
# use the index from the most common codes to select from the feature data
# the aggregation method and the columns to keep
agg_pcs_quantity = {unit_label:"sum", "quantity":"sum"}
groups = ["loc_date","date","code", "groupname"]
# make the range for one calendar year
start_date = "2020-04-01"
end_date = "2021-03-31"
# aggregate
m_common_m = fd[(fd.code.isin(fdx.most_common.index))].groupby(groups, as_index=False).agg(agg_pcs_quantity)
# set the index to the date column and sort values within the date rage
m_common_m.set_index("date", inplace=True)
m_common_m = m_common_m.sort_index().loc[start_date:end_date]
# set the order of the chart, group the codes by groupname columns and collect the respective object codes
an_order = m_common_m.groupby(["code","groupname"], as_index=False).quantity.sum().sort_values(by="groupname")["code"].values
# use the order array and resample each code for the monthly value
# store in a dict
mgr = {}
for a_code in an_order:
# resample by month
a_cell = m_common_m[(m_common_m.code==a_code)][unit_label].resample("M").mean().fillna(0)
a_cell = round(a_cell, 1)
this_group = {a_code:a_cell}
mgr.update(this_group)
# make df form dict and collect the abbreviated month name set that to index
by_month = pd.DataFrame.from_dict(mgr)
by_month["month"] = by_month.index.map(lambda x: get_month_names('abbreviated', locale=date_lang)[x.month])
by_month.set_index('month', drop=True, inplace=True)
# transpose to get months on the columns and set index to the object description
by_month = by_month.T
by_month["Objekt"] = by_month.index.map(lambda x: fdx.dMap.loc[x])
by_month.set_index("Objekt", drop=True, inplace=True)
mc_monthly_title = Paragraph("Häufigste Objekte im Monatsdurchschnitt", featuredata.subsection_title)
monthly_data_caption = f'Monatliches Durchschnittsergebnis der Erhebungen als {unit_label} der häufigsten Objekte für alle Erhebungsgebiete.'
figure_caption = Paragraph(monthly_data_caption, featuredata.caption_style)
# apply formatting to df for pdf figure/*
col_widths = [4.5*cm, *[1*cm]*(len(mc_comp.columns)-1)]
# make pdf table
col_widths = [4.5*cm, *[1.2*cm]*(len(mc_comp.columns)-1)]
monthly_table = featuredata.aSingleStyledTable(by_month, colWidths=col_widths, gradient=True)
monthly_table_caption = Paragraph(monthly_data_caption, new_caption_style)
monthly_table_and_caption = featuredata.tableAndCaption(monthly_table, monthly_table_caption, col_widths)
new_components = [
KeepTogether([
featuredata.large_space,
mc_monthly_title,
featuredata.large_space,
monthly_table_and_caption
])
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
# remove the index names for .html display
by_month.index.name = None
by_month.columns.name = None
aformatter = {x: featuredata.replaceDecimal for x in by_month.columns}
mcdm = by_month.style.format(aformatter).set_table_styles(heat_map_css_styles).background_gradient(axis=None, cmap="YlOrBr", vmin=by_month.min().min(), vmax=by_month.max().max())
glue(f'{this_feature["slug"]}_monthly_results_caption', monthly_data_caption, display=False)
glue(f'{this_feature["slug"]}_monthly_results', mcdm, display=False)
Apr. | Mai | Juni | Juli | Aug. | Sept. | Okt. | Nov. | Dez. | Jan. | Feb. | März | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
Wattestäbchen/Tupfer | 4,6 | 11,0 | 22,8 | 23,4 | 12,1 | 8,0 | 10,1 | 3,4 | 10,9 | 11,5 | 6,6 | 11,1 |
Kronkorken, Lasche von Dose/Ausfreisslachen | 3,6 | 4,9 | 7,8 | 7,3 | 4,8 | 3,0 | 2,5 | 1,5 | 2,2 | 3,1 | 9,4 | 2,5 |
Getränkeflaschen aus Glas, Glasfragmente | 2,5 | 13,3 | 10,8 | 11,2 | 11,9 | 24,4 | 20,9 | 8,2 | 22,8 | 22,1 | 18,3 | 14,6 |
Snack-Verpackungen | 29,5 | 18,0 | 37,5 | 38,0 | 21,7 | 31,9 | 20,8 | 11,2 | 12,1 | 17,6 | 20,9 | 46,6 |
Schaumstoffverpackungen/Isolierung | 1,1 | 4,8 | 21,7 | 30,8 | 36,4 | 14,7 | 10,4 | 12,7 | 7,4 | 6,6 | 4,0 | 8,4 |
Kunststoff-Bauabfälle | 14,5 | 10,6 | 7,6 | 11,5 | 8,9 | 5,2 | 5,9 | 2,4 | 5,8 | 7,2 | 6,9 | 8,4 |
Expandiertes Polystyrol | 42,2 | 32,3 | 73,7 | 55,1 | 66,2 | 44,7 | 51,7 | 77,1 | 23,2 | 17,6 | 22,7 | 23,4 |
Industriefolie (Kunststoff) | 29,7 | 15,4 | 20,5 | 29,9 | 17,0 | 25,0 | 16,9 | 10,8 | 7,7 | 14,2 | 18,0 | 46,2 |
Industriepellets (Nurdles) | 0,7 | 1,3 | 49,2 | 40,4 | 6,2 | 5,8 | 12,4 | 7,6 | 10,8 | 4,5 | 7,2 | 3,7 |
Styropor < 5mm | 1,1 | 2,9 | 11,1 | 24,9 | 4,6 | 4,7 | 3,4 | 19,0 | 10,2 | 6,2 | 13,7 | 1,5 |
Fragmentierte Kunststoffe | 43,8 | 50,5 | 147,8 | 100,1 | 89,4 | 66,3 | 36,2 | 22,7 | 71,4 | 50,1 | 28,1 | 47,6 |
Zigarettenfilter | 79,3 | 54,8 | 97,2 | 98,9 | 91,9 | 87,3 | 26,4 | 15,3 | 15,0 | 22,5 | 37,9 | 42,2 |
Abb. 1.8 #
Abbildung 1.8: Monatliches Durchschnittsergebnis der Erhebungen als p/100 m der häufigsten Objekte für alle Erhebungsgebiete.
1.4. Erhebungsergebnisse und Landnutzung#
Das Landnutzungsprofil ist eine Darstellung der Art und des Umfangs der wirtschaftlichen Aktivität und der Umweltbedingungen rund um den Erhebungsort. Die Schlüsselindikatoren aus den Ergebnissen der Datenerhebungen werden mit dem Landnutzungsprofil für einen Radius von 1500 m um den Erhebungsort verglichen.
Eine Assoziation ist eine Beziehung zwischen den Ergebnissen der Datenerhebungen und dem Landnutzungsprofil, die nicht auf Zufall beruht. Das Ausmass der Beziehung ist weder definiert noch linear.
Die Rangkorrelation ist ein nicht-parametrischer Test, um festzustellen, ob ein statistisch signifikanter Zusammenhang zwischen der Landnutzung und den bei einer Abfallobjekte-Erhebung identifizierten Objekten besteht.
Die verwendete Methode ist der Spearmans Rho oder Spearmans geordneter Korrelationskoeffizient. Die Testergebnisse werden bei p < 0,05 für alle gültigen Erhebungen an Seen im Untersuchungsgebiet ausgewertet.
Rot/Rosa steht für eine positive Assoziation
Gelb steht für eine negative Assoziation
Weiss bedeutet, dass keine statistische Grundlage für die Annahme eines Zusammenhangs besteht
Show code cell source
# add the river bassin to fd
# this needs to be updated directly
rb_map = admin_details.makeFeatureNameMap()
fd_copy = fd.copy()
fd_qq = fd[fd.water_name_slug == 'quatre-cantons'].copy()
fd_qq['water_name_slug'] = 'vierwaldstattersee'
fd = pd.concat([fd[fd.water_name_slug != 'quatre-cantons'], fd_qq])
fd["water_name"] = fd.water_name_slug.apply(lambda x: rb_map.loc[x])
corr_data = fd[(fd.code.isin(fdx.most_common.index))&(fd.water_name.isin(admin_details.lakes_of_interest))].copy()
land_use_columns = featuredata.default_land_use_columns
code_description_map = fdx.dMap
def make_plot_with_spearmans(data, ax, n, unit_label="p/100m"):
"""Gets Spearmans ranked correlation and make A/B scatter plot. Must proived a
matplotlib axis object.
"""
corr, a_p = stats.spearmanr(data[n], data[unit_label])
if a_p < 0.05:
if corr > 0:
ax.patch.set_facecolor("salmon")
ax.patch.set_alpha(0.5)
else:
ax.patch.set_facecolor("palegoldenrod")
ax.patch.set_alpha(0.5)
return ax, corr, a_p
# chart the results of test for association
fig, axs = plt.subplots(len(fdx.most_common.index),len(land_use_columns), figsize=(len(land_use_columns)*.5,len(fdx.most_common.index)*.5), sharey="row")
# the test is conducted on the survey results for each code
for i,code in enumerate(fdx.most_common.index):
# slice the data
data = corr_data[corr_data.code == code]
# run the test on for each land use feature
for j, n in enumerate(land_use_columns):
# assign ax and set some parameters
ax=axs[i, j]
ax.grid(False)
ax.tick_params(axis="both", which="both",bottom=False,top=False,labelbottom=False, labelleft=False, left=False)
# check the axis and set titles and labels
if i == 0:
ax.set_title(f"{featuredata.luse_de[n]}", rotation=90, ha="left", fontsize=12)
else:
pass
if j == 0:
ax.set_ylabel(f"{code_description_map[code]}", rotation=0, ha="right", labelpad=10, fontsize=12)
ax.set_xlabel(" ")
else:
ax.set_xlabel(" ")
ax.set_ylabel(" ")
# run test
ax = make_plot_with_spearmans(data, ax, n, unit_label=unit_label)
plt.subplots_adjust(wspace=0, hspace=0)
# figure caption
caption_spearmans = [
"Ausgewertete Korrelationen der am häufigsten gefundenen Objekte in Bezug auf das Landnutzungsprofil ",
"im Erhebungsgebiet all. Für alle gültigen Erhebungen an Seen n=118. Legende: wenn p > 0,05 = weiss, ",
"wenn p < 0,05 und Rho > 0 = rot, wenn p < 0,05 und Rho < 0 = gelb.",
]
spearmans = ''.join(caption_spearmans)
figure_name = f'{this_feature["slug"]}_survey_area_spearmans'
sample_summaries_file_name = f'{save_fig_prefix}{figure_name}.jpeg'
save_figure_kwargs.update({"fname":sample_summaries_file_name})
plt.savefig(**save_figure_kwargs)
glue(f'{this_feature["slug"]}_spearmans_caption', spearmans, display=False)
glue(f'{this_feature["slug"]}_survey_area_spearmans', fig, display=False)
plt.close()
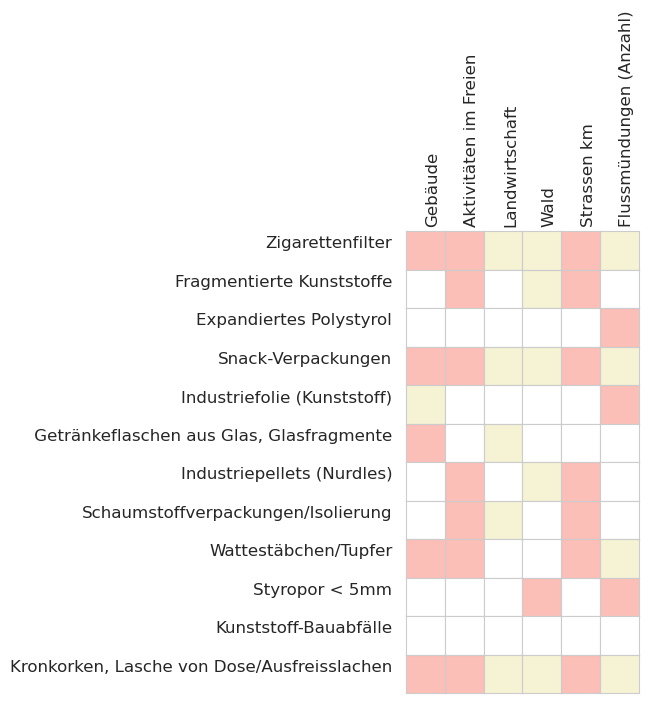
Abb. 1.9 #
Abbildung 1.9: Ausgewertete Korrelationen der am häufigsten gefundenen Objekte in Bezug auf das Landnutzungsprofil im Erhebungsgebiet all. Für alle gültigen Erhebungen an Seen n=118. Legende: wenn p > 0,05 = weiss, wenn p < 0,05 und Rho > 0 = rot, wenn p < 0,05 und Rho < 0 = gelb.
1.5. Verwendungszweck der gefundenen Objekte#
Der Verwendungszweck basiert auf der Verwendung des Objekts, bevor es weggeworfen wurde, oder auf der Artikelbeschreibung, wenn die ursprüngliche Verwendung unbestimmt ist. Identifizierte Objekte werden einer der 260 vordefinierten Kategorien zugeordnet. Die Kategorien werden je nach Verwendung oder Artikelbeschreibung gruppiert.
Abwasser: Objekte, die aus Kläranlagen freigesetzt werden, sprich Objekte, die wahrscheinlich über die Toilette entsorgt werden
Mikroplastik (< 5 mm): fragmentierte Kunststoffe und Kunststoffharze aus der Vorproduktion
Infrastruktur: Artikel im Zusammenhang mit dem Bau und der Instandhaltung von Gebäuden, Strassen und der Wasser-/Stromversorgung
Essen und Trinken: alle Materialien, die mit dem Konsum von Essen und Trinken in Zusammenhang stehen
Landwirtschaft: Materialien z. B. für Mulch und Reihenabdeckungen, Gewächshäuser, Bodenbegasung, Ballenverpackungen. Einschliesslich Hartkunststoffe für landwirtschaftliche Zäune, Blumentöpfe usw.
Tabakwaren: hauptsächlich Zigarettenfilter, einschliesslich aller mit dem Rauchen verbundenen Materialien
Freizeit und Erholung: Objekte, die mit Sport und Freizeit zu tun haben, z. B. Angeln, Jagen, Wandern usw.
Verpackungen ausser Lebensmittel und Tabak: Verpackungsmaterial, das nicht lebensmittel- oder tabakbezogen ist
Plastikfragmente: Plastikteile unbestimmter Herkunft oder Verwendung
Persönliche Gegenstände: Accessoires, Hygieneartikel und Kleidung
Im Anhang (Kapitel 1.8.3) befindet sich die vollständige Liste der identifizierten Objekte, einschliesslich Beschreibungen und Gruppenklassifizierung. Das Kapitel Codegruppen beschreibt jede Codegruppe im Detail und bietet eine umfassende Liste aller Objekte in einer Gruppe.
Show code cell source
components = fdx.componentCodeGroupResults()
# pivot that
pt_comp = components[["river_bassin", "groupname", '% of total' ]].pivot(columns="river_bassin", index="groupname")
# quash the hierarchal column index
pt_comp.columns = pt_comp.columns.get_level_values(1)
pt_comp.rename(columns = proper_column_names, inplace=True)
# the aggregated totals for the period
pt_period = period_data.parentGroupTotals(parent=False, percent=True)
pt_comp[top] = pt_period
# caption
code_group_percent_caption = ['Prozentuale Verteilung der identifizierten Objekte nach Verwendungszweck.']
code_group_percent_caption = ''.join(code_group_percent_caption)
# format for data frame
pt_comp.index.name = None
pt_comp.columns.name = None
aformatter = {x: '{:.0%}' for x in pt_comp.columns}
ptd = pt_comp.style.format(aformatter).set_table_styles(heat_map_css_styles).background_gradient(axis=None, vmin=pt_comp.min().min(), vmax=pt_comp.max().max(), cmap="YlOrBr")
ptd = ptd.applymap_index(featuredata.rotateText, axis=1)
# the caption prefix is used in the case where the table needs to be split horzontally
caption_prefix = 'Verwendungszweck oder Beschreibung der identifizierten Objekte in % der Gesamtzahl nach Gemeinden: '
col_widths = [4.5*cm, *[1.2*cm]*(len(pt_comp.columns)-1)]
cgpercent_tables = splitTableWidth(pt_comp, gradient=True, caption_prefix=caption_prefix, caption= code_group_percent_caption,
this_feature=this_feature["name"], vertical_header=True, colWidths=col_widths)
glue(f'{this_feature["slug"]}_codegroup_percent_caption', code_group_percent_caption, display=False)
glue(f'{this_feature["slug" ]}_codegroup_percent', ptd, display=False)
Aare | Linth | Rhone | Ticino | Alle Erhebungsgebiete | |
---|---|---|---|---|---|
Abwasser | 4% | 2% | 6% | 2% | 5% |
Essen und Trinken | 19% | 22% | 18% | 21% | 19% |
Freizeit und Erholung | 5% | 5% | 4% | 2% | 4% |
Infrastruktur | 13% | 15% | 22% | 16% | 18% |
Landwirtschaft | 7% | 6% | 5% | 4% | 6% |
Mikroplastik (< 5mm) | 5% | 3% | 10% | 10% | 8% |
Persönliche Gegenstände | 3% | 4% | 2% | 3% | 3% |
Plastikfragmente | 14% | 10% | 15% | 9% | 14% |
Tabakwaren | 20% | 24% | 12% | 26% | 17% |
Verpackungen ohne Lebensmittel/Tabak | 7% | 7% | 4% | 6% | 5% |
nicht klassifiziert | 1% | 1% | 1% | 0% | 1% |
Abb. 1.10 #
Abbildung 1.10: Prozentuale Verteilung der identifizierten Objekte nach Verwendungszweck.
Show code cell source
# pivot that
grouppcs_comp = components[["river_bassin", "groupname", unit_label ]].pivot(columns="river_bassin", index="groupname")
# quash the hierarchal column index
grouppcs_comp.columns = grouppcs_comp.columns.get_level_values(1)
grouppcs_comp.rename(columns = proper_column_names, inplace=True)
# the aggregated totals for the period
pt_period = period_data.parentGroupTotals(parent=False, percent=False)
grouppcs_comp[top] = pt_period
# color gradient of restults
code_group_pcsm_gradient = featuredata.colorGradientTable(grouppcs_comp)
grouppcs_comp.index.name = None
grouppcs_comp.columns.name = None
# pdf and display output
code_group_pcsm_caption = [
"Das Erhebungsgebiet Rhône weist die höchsten Medianwerte für ",
"die jeweiligen Verwendungszwecke auf. Allerdings ist der prozentuale ",
"Anteil von Objekten, die mit Tabakwaren, Essen und Trinken zu tun haben, ",
"geringer als der von Objekten, die mit der Infrastruktur zu tun haben."
]
code_group_pcsm_caption = ''.join(code_group_pcsm_caption)
caption_prefix = f'Verwendungszweck der gefundenen Objekte Median {unit_label} am '
col_widths = [4.5*cm, *[1.2*cm]*(len(grouppcs_comp.columns)-1)]
cgpcsm_tables = splitTableWidth(grouppcs_comp, gradient=True, caption_prefix=caption_prefix, caption=code_group_pcsm_caption,
this_feature=this_feature["name"], vertical_header=True, colWidths=col_widths)
if isinstance(cgpcsm_tables, (list, np.ndarray)):
new_components = [
featuredata.large_space,
*cgpercent_tables,
featuredata.larger_space,
*cgpcsm_tables,
featuredata.larger_space
]
else:
new_components = [
featuredata.large_space,
cgpercent_tables,
featuredata.larger_space,
cgpcsm_tables,
featuredata.larger_space
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
aformatter = {x: featuredata.replaceDecimal for x in grouppcs_comp.columns}
cgp = grouppcs_comp.style.format(aformatter).set_table_styles(heat_map_css_styles).background_gradient(axis=None, vmin=grouppcs_comp.min().min(), vmax=grouppcs_comp.max().max(), cmap="YlOrBr")
cgp= cgp.applymap_index(featuredata.rotateText, axis=1)
glue(f'{this_feature["slug" ]}_codegroup_pcsm_caption', code_group_pcsm_caption, display=False)
glue(f'{this_feature["slug" ]}_codegroup_pcsm', cgp, display=False)
Aare | Linth | Rhone | Ticino | Alle Erhebungsgebiete | |
---|---|---|---|---|---|
Abwasser | 3,0 | 0,5 | 19,0 | 2,0 | 3,0 |
Essen und Trinken | 27,0 | 30,5 | 76,0 | 29,0 | 37,0 |
Freizeit und Erholung | 6,0 | 4,0 | 16,5 | 3,5 | 6,0 |
Infrastruktur | 15,0 | 12,5 | 55,5 | 22,5 | 20,0 |
Landwirtschaft | 6,0 | 3,0 | 14,0 | 6,0 | 7,0 |
Mikroplastik (< 5mm) | 1,0 | 0,0 | 11,5 | 0,0 | 1,0 |
Persönliche Gegenstände | 4,0 | 4,0 | 10,0 | 6,5 | 6,0 |
Plastikfragmente | 18,5 | 10,5 | 48,0 | 9,5 | 18,0 |
Tabakwaren | 15,0 | 26,5 | 50,0 | 18,0 | 25,0 |
Verpackungen ohne Lebensmittel/Tabak | 7,5 | 10,0 | 13,0 | 5,5 | 9,0 |
nicht klassifiziert | 0,0 | 0,0 | 2,0 | 0,0 | 0,0 |
Abb. 1.11 #
Abbildung 1.11: Das Erhebungsgebiet Rhône weist die höchsten Medianwerte für die jeweiligen Verwendungszwecke auf. Allerdings ist der prozentuale Anteil von Objekten, die mit Tabakwaren, Essen und Trinken zu tun haben, geringer als der von Objekten, die mit der Infrastruktur zu tun haben.
1.6. Fliessgewässer#
Show code cell source
# summary of sample totals
rivers = fdr.sample_totals
lakes = fdx.sample_totals
csx = fdr.sample_summary.copy()
combined_summary =[(x, featuredata.thousandsSeparator(int(csx[x]), language)) for x in csx.index]
# make the charts
fig = plt.figure(figsize=(10,6))
aspec = fig.add_gridspec(ncols=11, nrows=3)
ax = fig.add_subplot(aspec[:, :6])
line_label = F"{rate} median:{top}"
sns.scatterplot(data=lakes, x="date", y=unit_label, color="black", alpha=0.4, label="Seen", ax=ax)
sns.scatterplot(data=rivers, x="date", y=unit_label, color="red", s=34, ec="white",label="Fliessgewässer", ax=ax)
ax.set_ylabel(unit_label, labelpad=10, fontsize=14)
ax.set_xlabel("")
ax.xaxis.set_minor_locator(days)
ax.xaxis.set_major_formatter(months_fmt)
# ax.margins(x=.05, y=.05)
ax.set_ylim(-50, 2000)
a_col = [this_feature["name"], "total"]
axone = fig.add_subplot(aspec[:, 6:])
sut.hide_spines_ticks_grids(axone)
table_five = sut.make_a_table(axone, combined_summary, colLabels=a_col, colWidths=[.75,.25], bbox=[0,0,1,1], **{"loc":"lower center"})
table_five.get_celld()[(0,0)].get_text().set_text(" ")
table_five.set_fontsize(12)
rivers_caption = [
"Links: Gesamtergebnisse der Erhebungen an Fliessgewässern für alle Erhebungsgebiete von ",
f"März 2020 bis Mai 2021, n=55. Werte über {'2000 '}{unit_label} m sind nicht dargestellt. Rechts: ",
"Zusammenfassende Daten zu Fliessgewässern."
]
rivers_caption = ''.join(rivers_caption)
figure_name = f'{this_feature["slug"]}river_sample_totals'
river_totals_file_name = f'{save_fig_prefix}{figure_name}.jpeg'
save_figure_kwargs.update({"fname":river_totals_file_name})
plt.tight_layout()
plt.savefig(**save_figure_kwargs)
glue(f'{this_feature["slug" ]}_survey_area_rivers_summary_caption', rivers_caption, display=False)
glue(f'{this_feature["slug" ]}_survey_area_rivers_summary', fig, display=False)
plt.close()
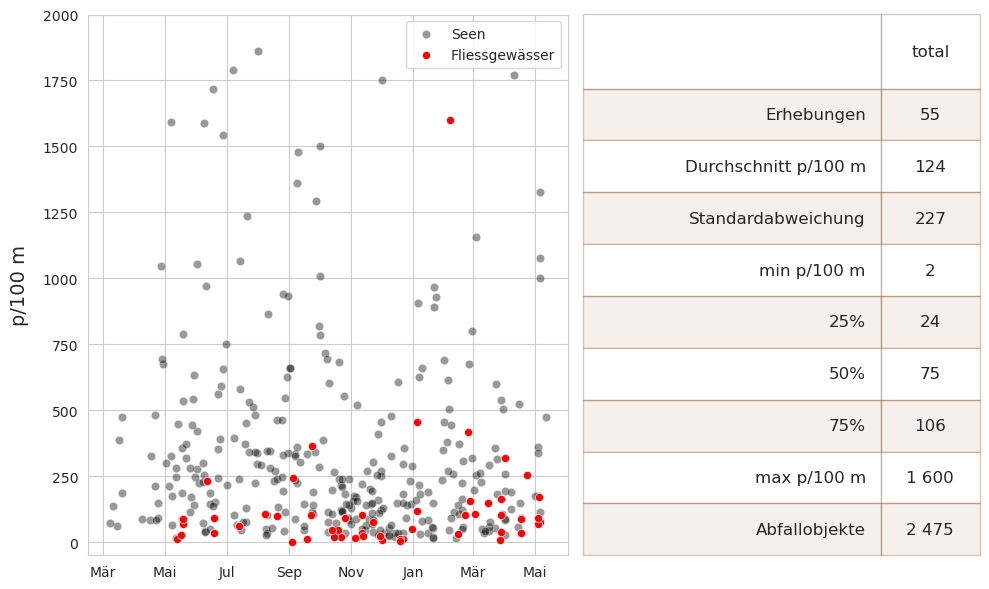
Abb. 1.12 #
Abbildung 1.12: Links: Gesamtergebnisse der Erhebungen an Fliessgewässern für alle Erhebungsgebiete von März 2020 bis Mai 2021, n=55. Werte über 2000 p/100 m m sind nicht dargestellt. Rechts: Zusammenfassende Daten zu Fliessgewässern.
Die an Fliessgewässern am häufigsten gefundenen Objekte
Show code cell source
# the most common objects results
rmost_common_display = fdr.most_common.copy()
cols_to_use = featuredata.most_common_objects_table_de
cols_to_use.update({unit_label:unit_label})
rmost_common_display.rename(columns=cols_to_use, inplace=True)
rmost_common_display = rmost_common_display[cols_to_use.values()].copy()
rmost_common_display.set_index("Objekte", drop=True, inplace=True)
# format the data for .pdf
data = rmost_common_display.copy()
data["Anteil"] = data["Anteil"].map(lambda x: f"{int(x)}%")
data['Objekte (St.)'] = data['Objekte (St.)'].map(lambda x:featuredata.thousandsSeparator(x, language))
data['Häufigkeitsrate'] = data['Häufigkeitsrate'].map(lambda x: f"{x}%")
data[unit_label] = data[unit_label].map(lambda x: featuredata.replaceDecimal(round(x,1)))
# make caption text
mc_caption_string = [
"Die häufigsten Objekte aus Erhebungen an Fliessgewässern. Windeln, Feuchttücher und Taschen/Tüten aus Kunststoff resp. ",
"Fragmente davon gehören nur entlang von Fliessgewässern zu den häufigsten Objekten, nicht jedoch an Ufern von Seen. "
]
mc_caption_string = ''.join(mc_caption_string)
# make .pdf table and caption
col_widths = [4.5*cm, 2.2*cm, 2*cm, 2.8*cm, 2*cm]
r_mc_table = featuredata.aSingleStyledTable(data, colWidths=col_widths)
r_mc_table_caption = Paragraph(mc_caption_string, new_caption_style)
r_t_and_c = featuredata.tableAndCaption(r_mc_table, r_mc_table_caption, col_widths)
rmost_common_display.index.name = None
rmost_common_display.columns.name = None
# set pandas display
aformatter = {
"Anteil":lambda x: f"{int(x)}%",
f"{unit_label}": lambda x: featuredata.replaceDecimal(x, "de"),
"Häufigkeitsrate": lambda x: f"{int(x)}%",
"Objekte (St.)": lambda x: featuredata.thousandsSeparator(int(x), "de")
}
mcd = rmost_common_display.style.format(aformatter).set_table_styles(table_css_styles)
glue(f'{this_feature["slug" ]}_rivers_most_common_caption', mc_caption_string, display=False)
glue(f'{this_feature["slug" ]}_rivers_most_common_tables', mcd, display=False)
Objekte (St.) | Anteil | Häufigkeitsrate | p/100 m | |
---|---|---|---|---|
Windeln – Feuchttücher | 305 | 12% | 23% | 0,0 |
Zigarettenfilter | 300 | 12% | 63% | 4,0 |
Getränkeflaschen aus Glas, Glasfragmente | 181 | 7% | 34% | 0,0 |
Industriefolie (Kunststoff) | 176 | 7% | 40% | 0,0 |
Snack-Verpackungen | 130 | 5% | 61% | 2,0 |
Fragmentierte Kunststoffe | 109 | 4% | 54% | 2,0 |
Verpackungsfolien, nicht für Lebensmittel | 87 | 3% | 29% | 0,0 |
Kronkorken, Lasche von Dose/Ausfreisslachen | 80 | 3% | 32% | 0,0 |
Einkaufstaschen, Shoppingtaschen | 60 | 2% | 21% | 0,0 |
Expandiertes Polystyrol | 53 | 2% | 20% | 0,0 |
Abb. 1.13 #
Abbildung 1.13: Die häufigsten Objekte aus Erhebungen an Fliessgewässern. Windeln, Feuchttücher und Taschen/Tüten aus Kunststoff resp. Fragmente davon gehören nur entlang von Fliessgewässern zu den häufigsten Objekten, nicht jedoch an Ufern von Seen.
1.7. Anhang#
1.7.1. Schaumstoffe und Kunststoffe nach Grösse#
Die folgende Tabelle enthält die Komponenten «Gfoam» und «Gfrags», die für die Analyse gruppiert wurden. Objekte, die als Schaumstoffe gekennzeichnet sind, werden als Gfoam gruppiert und umfassen alle geschäumten Polystyrol-Kunststoffe > 0,5 cm. Kunststoffteile und Objekte aus kombinierten Kunststoff- und Schaumstoffmaterialien > 0,5 cm werden für die Analyse als Gfrags gruppiert.
Show code cell source
annex_title = Paragraph("Anhang", featuredata.section_title)
frag_sub_title = Paragraph("Schaumstoffe und Kunststoffe nach Grösse", featuredata.subsection_title)
frag_paras = [
"Die folgende Tabelle enthält die Komponenten «Gfoam» und «Gfrag», die für die Analyse gruppiert wurden. ",
"Objekte, die als Schaumstoffe gekennzeichnet sind, werden als Gfoam gruppiert und umfassen alle geschäumten ",
"Polystyrol-Kunststoffe > 0,5 cm. Kunststoffteile und Objekte aus kombinierten Kunststoff - und Schaumstoffmaterialien > 0,5 ",
"cm werden für die Analyse als Gfrags gruppiert."
]
frag_p = "".join(frag_paras)
frag = Paragraph(frag_p, featuredata.p_style)
frag_caption = [
"Fragmentierte Kunststoffe und geschäumte Kunststoffe verschiedener ",
f"Grössenordnungen nach Median {unit_label}, Anzahl der gefundenen Objekte ",
"und prozentualer Anteil an der Gesamtmenge."
]
frag_captions = ''.join(frag_caption)
# collect the data before aggregating foams for all locations in the survye area
# group by loc_date and code
# Combine the different sizes of fragmented plastics and styrofoam
# the codes for the foams
before_agg = pd.read_csv("resources/checked_before_agg_sdata_eos_2020_21.csv")
some_foams = ["G81", "G82", "G83", "G74"]
before_agg.rename(columns={"p/100m":unit_label}, inplace=True)
# the codes for the fragmented plastics
some_frag_plas = list(before_agg[before_agg.groupname == "plastic pieces"].code.unique())
mask = ((before_agg.code.isin([*some_frag_plas, *some_foams]))&(before_agg.location.isin(admin_summary["locations_of_interest"])))
agg_pcs_median = {unit_label:"median", "quantity":"sum"}
fd_frags_foams = before_agg[mask].groupby(["loc_date","code"], as_index=False).agg(agg_pcs_quantity)
fd_frags_foams = fd_frags_foams.groupby("code").agg(agg_pcs_median)
fd_frags_foams["item"] = fd_frags_foams.index.map(lambda x: fdx.dMap.loc[x])
fd_frags_foams["% of total"] = (fd_frags_foams.quantity/fd.quantity.sum()).round(2)
# table data
data = fd_frags_foams[["item",unit_label, "quantity", "% of total"]]
data.rename(columns={"item":"Objekt", "quantity":"Objekte (St.)", "% of total":"Anteil"}, inplace=True)
data.set_index("Objekt", inplace=True, drop=True)
data.index.name = None
aformatter = {
f"{unit_label}": lambda x: featuredata.replaceDecimal(x, "de"),
"Objekte (St.)": lambda x: featuredata.thousandsSeparator(int(x), "de"),
"Anteil":'{:.0%}',
}
frags_table = data.style.format(aformatter).set_table_styles(table_css_styles)
glue("all_frag_table_caption", frag_captions, display=False)
glue("all_frags_table", frags_table, display=False)
col_widths = [7*cm, *[2*cm]*(len(dims_table.columns)-1)]
frag_table = featuredata.aSingleStyledTable(data, colWidths=col_widths)
frags_table_caption = Paragraph(frag_captions, new_caption_style)
frag_table_and_caption = featuredata.tableAndCaption(frag_table, frags_table_caption, col_widths)
new_components = [
KeepTogether([
annex_title,
featuredata.small_space,
frag_sub_title,
featuredata.smaller_space,
frag,
featuredata.small_space
]),
frag_table_and_caption
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
p/100 m | Objekte (St.) | Anteil | |
---|---|---|---|
Schaumstoffverpackungen/Isolierung | 0,0 | 203 | 0% |
Objekte aus Kunststoff/Polystyrol 0,5 - 2,5 cm | 0,0 | 495 | 1% |
Objekte aus Kunststoff/Polystyrol 2,5 - 50 cm | 0,0 | 111 | 0% |
Objekte aus Kunststoff 0,5 - 2,5 cm | 7,0 | 3 662 | 7% |
Objekte aus Kunststoff 2,5 - 50 cm | 7,0 | 3 130 | 6% |
Objekte aus Kunststoff > 50 cm | 0,0 | 2 | 0% |
Objekte aus expandiertem Polystyrol 0,5 - 2,5 cm | 2,0 | 3 713 | 7% |
Objekte aus expandiertem Polystyrol 2,5 - 50 cm | 1,0 | 1 843 | 3% |
Objekte aus expandiertem Polystyrol > 50 cm | 0,0 | 7 | 0% |
Abb. 1.14 #
Abbildung 1.14: Fragmentierte Kunststoffe und geschäumte Kunststoffe verschiedener Grössenordnungen nach Median p/100 m, Anzahl der gefundenen Objekte und prozentualer Anteil an der Gesamtmenge.
1.7.2. Beteiligte Organisationen:#
Precious Plastic Léman
Association pour la Sauvegarde du Léman
Geneva international School
Teilnehmende am Kurs zu Abfalltechnik (Solid waste engineering), Eidgenössische Technische Hochschule Lausanne
Summit Foundation
Ostschweizer Fachhochschule OST
Hackuarium
hammerdirt
Show code cell source
# make the organisation list for the .pdf
some_list_items = [
"Precious Plastic Léman",
"Association pour la Sauvegarde du Léman",
"Geneva international School",
"Teilnehmende am Kurs zu Abfalltechnik (Solid waste engineering), Eidgenössische Technische Hochschule Lausanne",
"Summit Foundation",
"Ostschweizer Fachhochschule OST",
"Hackuarium",
"hammerdirt"
]
organisation_list = makeAList(some_list_items)
organisation_title = Paragraph("Organisationen:", featuredata.subsection_title)
new_components = [
featuredata.large_space,
organisation_title,
featuredata.small_space,
organisation_list
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
1.7.3. Die Erhebungsorte#
Show code cell source
# display the survey locations
pd.set_option("display.max_rows", None)
disp_columns = ["latitude", "longitude", "city"]
disp_beaches = admin_details.df_beaches.loc[admin_summary["locations_of_interest"]][disp_columns]
disp_beaches.reset_index(inplace=True)
disp_beaches.rename(columns={"city":"stat", "slug":"standort"}, inplace=True)
disp_beaches.set_index("standort", inplace=True, drop=True)
def aStyledTableWithTitleRow(data, header_style: Paragraph = featuredata.styled_table_header, title: str = None,
data_style: Paragraph = featuredata.table_style_centered, colWidths: list = None, style: list = None):
table_data = data.reset_index()
headers = [Paragraph(str(x), header_style) for x in data.columns]
headers = [Paragraph(" ", data_style) , *headers]
if style is None:
style = featuredata.default_table_style
new_rows = []
for a_row in table_data.values.tolist():
if isinstance(a_row[0], str):
row_index = Paragraph(a_row[0], featuredata.table_style_right)
row_data = [Paragraph(str(x), data_style) for x in a_row[1:]]
new_row = [row_index, *row_data]
new_rows.append(new_row)
else:
row_data = [Paragraph(str(x), data_style) for x in a_row[1:]]
new_rows.append(row_data)
table_title_style = [
('FONTNAME', (0,0), (-1,-1), 'Helvetica'),
('FONTSIZE', (0, 0), (-1, -1), 12),
('ROWBACKGROUND', (0,0), (-1,-1), [colors.white]),
('TOPPADDING', (0, 0), (-1, -1), 3),
('BOTTOMPADDING', (0, 0), (-1, -1), 3)
]
table_title = Table([[title]], style=table_title_style, colWidths=sum(colWidths))
new_table = [[table_title], headers, *new_rows]
table = Table(new_table, style=style, colWidths=colWidths, repeatRows=2)
return table
# make this into a pdf table
location_subsection = Paragraph("Die Erhebungsorte und Inventar der Objekte", featuredata.subsection_title)
col_widths = [6*cm, 2.2*cm, 2.2*cm, 3*cm]
pdf_table = aStyledTableWithTitleRow(disp_beaches, title="Die Erhebungsorte", colWidths=col_widths)
disp_beaches
latitude | longitude | stat | |
---|---|---|---|
standort | |||
maladaire | 46.446296 | 6.876960 | La Tour-de-Peilz |
preverenges | 46.512690 | 6.527657 | Préverenges |
caprino | 45.987963 | 8.986241 | Lugano |
foce-del-cassarate | 46.002411 | 8.961477 | Lugano |
lido | 46.002004 | 8.962156 | Lugano |
lugano-centro | 46.002627 | 8.950724 | Lugano |
spiaggia-parco-ciani | 46.002510 | 8.960820 | Lugano |
via-brunari-spiaggia | 46.202350 | 9.016910 | Bellinzona |
golene-gudo | 46.170655 | 8.946657 | Bellinzona |
isole-di-brissago-porto | 46.132760 | 8.735030 | Brissago |
magadino-lido | 46.149349 | 8.855663 | Gambarogno |
rivapiana | 46.172079 | 8.813255 | Minusio |
via-mirasole-spiaggia | 46.199530 | 9.014930 | Bellinzona |
aare-port | 47.116170 | 7.269550 | Port |
limmat_dietikon_keiserp | 47.407989 | 8.409540 | Dietikon |
schutzenmatte | 47.057666 | 7.634001 | Burgdorf |
zugerseecholler_cham_blarerm | 47.178216 | 8.480013 | Zug |
la-petite-plage | 46.785054 | 6.656877 | Yverdon-les-Bains |
vidy-ruines | 46.516221 | 6.596279 | Lausanne |
baby-plage-geneva | 46.208558 | 6.162923 | Genève |
grand-clos | 46.387746 | 6.843686 | Saint-Gingolph |
weissenau-neuhaus | 46.676583 | 7.817528 | Unterseen |
evole-plage | 46.989477 | 6.923920 | Neuchâtel |
oberi-chlihochstetten | 46.896025 | 7.532114 | Rubigen |
plage-de-serriere | 46.984850 | 6.913450 | Neuchâtel |
mullermatte | 47.133339 | 7.227907 | Biel/Bienne |
limmat_unterengstringen_oggierbuhrer | 47.409400 | 8.446933 | Unterengstringen |
limmat_zuerich_wagnerc | 47.403496 | 8.477770 | Zürich |
limmat_zurich_mortensena_meiera | 47.400252 | 8.485411 | Zürich |
quai-maria-belgia | 46.460156 | 6.836718 | Vevey |
sihl_zuerich_eggerskoehlingera | 47.381898 | 8.538328 | Zürich |
bielersee_vinelz_fankhausers | 47.038398 | 7.108311 | Vinelz |
erlach-camping-strand | 47.047159 | 7.097854 | Erlach |
anarchy-beach | 46.447216 | 6.859612 | La Tour-de-Peilz |
gasi-strand | 47.128442 | 9.110831 | Weesen |
rastplatz-stampf | 47.215177 | 8.844286 | Rapperswil-Jona |
zuerichsee_richterswil_benkoem_2 | 47.217646 | 8.698713 | Richterswil |
sentiero-giro-del-golf-spiaggia | 46.145770 | 8.790430 | Ascona |
vira-gambarogno | 46.143343 | 8.838759 | Gambarogno |
canale-saleggi | 46.200625 | 9.015853 | Bellinzona |
gummligrabbe | 46.989290 | 7.250730 | Kallnach |
mannewil | 46.996382 | 7.239024 | Kallnach |
lavey-les-bains-2 | 46.207726 | 7.011685 | Lavey-Morcles |
schusspark-strand | 47.146500 | 7.268620 | Biel/Bienne |
walensee_walenstadt_wysse | 47.121828 | 9.299552 | Walenstadt |
pfafikon-bad | 47.206766 | 8.774182 | Freienbach |
zurichsee_kusnachterhorn_thirkell-whitej | 47.317685 | 8.576860 | Küsnacht (ZH) |
plage-de-cheyres | 46.818689 | 6.782256 | Cheyres-Châbles |
leuk-mattenstrasse | 46.314754 | 7.622521 | Leuk |
thun-strandbad | 46.739939 | 7.633520 | Thun |
zurichsee_wollishofen_langendorfm | 47.345890 | 8.536155 | Zürich |
pont-sous-terre | 46.202960 | 6.131577 | Genève |
oben-am-see | 46.744451 | 8.049921 | Brienz (BE) |
thunersee_spiez_meierd_1 | 46.704437 | 7.657882 | Spiez |
cully-plage | 46.488887 | 6.741396 | Bourg-en-Lavaux |
preverenges-le-sout | 46.508905 | 6.534526 | Préverenges |
la-pecherie | 46.463919 | 6.385732 | Allaman |
villa-barton | 46.222350 | 6.152500 | Genève |
untertenzen | 47.115260 | 9.254780 | Quarten |
zurcher-strand | 47.364200 | 8.537420 | Zürich |
oyonne | 46.456682 | 6.852262 | La Tour-de-Peilz |
lavey-la-source | 46.200804 | 7.021866 | Lavey-Morcles |
lavey-les-bains | 46.205159 | 7.012722 | Lavey-Morcles |
spackmatt | 47.223749 | 7.576711 | Luterbach |
luscherz-plage | 47.047955 | 7.151242 | Lüscherz |
strandboden-biel | 47.132510 | 7.233142 | Biel/Bienne |
jona-river-rastplatz | 47.216100 | 8.844430 | Rapperswil-Jona |
reuss_hunenberg_eberhardy | 47.232976 | 8.401012 | Hünenberg |
reuss_ottenbach_schoenenbergerl | 47.278354 | 8.394224 | Merenschwand |
zuerichsee_staefa_hennm | 47.234643 | 8.769881 | Stäfa |
signalpain | 46.786200 | 6.647360 | Yverdon-les-Bains |
baby-plage-ii-geneve | 46.208940 | 6.164330 | Genève |
pointe-dareuse | 46.946190 | 6.870970 | Boudry |
les-glariers | 46.176736 | 7.228925 | Riddes |
nidau-strand | 47.127196 | 7.232613 | Nidau |
tiger-duck-beach | 46.518256 | 6.582546 | Saint-Sulpice (VD) |
linthdammweg | 47.131790 | 9.091910 | Weesen |
mols-rocks | 47.114343 | 9.288174 | Quarten |
seeflechsen | 47.130223 | 9.103400 | Glarus Nord |
seemuhlestrasse-strand | 47.128640 | 9.295100 | Walenstadt |
muhlehorn-dorf | 47.118448 | 9.172124 | Glarus Nord |
zuerichsee_waedenswil_colomboc_1 | 47.219547 | 8.691961 | Richterswil |
camp-des-peches | 47.052812 | 7.074053 | Le Landeron |
delta-park | 46.720078 | 7.635304 | Spiez |
le-pierrier | 46.439727 | 6.888968 | Montreux |
pecos-plage | 46.803590 | 6.636650 | Grandson |
beich | 47.048495 | 7.200528 | Walperswil |
aare_suhrespitz_badert | 47.405669 | 8.066018 | Aarau |
tschilljus | 46.304399 | 7.580262 | Salgesch |
sundbach-strand | 46.684386 | 7.794768 | Beatenberg |
wycheley | 46.740370 | 8.048640 | Brienz (BE) |
aare_koniz_hoppej | 46.934588 | 7.458170 | Köniz |
camping-gwatt-strand | 46.727140 | 7.629620 | Thun |
bern-tiergarten | 46.933157 | 7.452004 | Bern |
boiron | 46.491030 | 6.480162 | Tolochenaz |
rocky-plage | 46.209737 | 6.164952 | Genève |
lacleman_gland_lecoanets | 46.402811 | 6.281959 | Gland |
route-13 | 46.162521 | 8.782579 | Locarno |
murg-bad | 47.115307 | 9.215691 | Quarten |
schmerikon-bahnhof-strand | 47.224780 | 8.944180 | Schmerikon |
aabach | 47.220989 | 8.940365 | Schmerikon |
la-thiele_le-mujon_confluence | 46.775340 | 6.630900 | Yverdon-les-Bains |
nouvelle-plage | 46.856646 | 6.848428 | Estavayer |
baye-de-montreux-g | 46.430834 | 6.908778 | Montreux |
les-vieux-ronquoz | 46.222049 | 7.361664 | Sion |
sihl_zuerich_eichenbergerd | 47.339588 | 8.516697 | Zürich |
versoix | 46.289194 | 6.170569 | Versoix |
augustmutzenbergstrandweg | 46.686640 | 7.689760 | Spiez |
parc-des-pierrettes | 46.515215 | 6.575531 | Saint-Sulpice (VD) |
plage-de-st-sulpice | 46.513265 | 6.570977 | Saint-Sulpice (VD) |
aare_bern_gerberm | 46.989363 | 7.452098 | Bern |
brugg-be-buren-bsg-standort | 47.122220 | 7.281410 | Brügg |
kusnachter-dorfbach-tobelweg-1-4 | 47.317770 | 8.588258 | Küsnacht (ZH) |
ruisseau-de-la-croix-plage | 46.813920 | 6.774700 | Cheyres-Châbles |
ligerz-strand | 47.083979 | 7.135894 | Ligerz |
hafeli | 46.690283 | 7.898592 | Bönigen |
aare-solothurn-lido-strand | 47.196949 | 7.521643 | Solothurn |
bern-fahrstrasse | 46.975866 | 7.444210 | Bern |
gals-reserve | 47.046272 | 7.085007 | Gals |
ascona-traghetto-spiaggia | 46.153882 | 8.768480 | Ascona |
pacha-mama-gerra | 46.123473 | 8.783508 | Gambarogno |
gerra-gambarogno | 46.123366 | 8.785786 | Gambarogno |
san-nazzaro-gambarogno | 46.130443 | 8.798657 | Gambarogno |
hauterive-petite-plage | 47.010797 | 6.980304 | Hauterive (NE) |
flibach-river-right-bank | 47.133742 | 9.105461 | Weesen |
aare-limmatspitz | 47.501060 | 8.237371 | Gebenstorf |
aare_brugg_buchie | 47.494855 | 8.236558 | Brugg |
linth_route9brucke | 47.125730 | 9.117340 | Glarus Nord |
seez_spennwiesenbrucke | 47.114948 | 9.310123 | Walenstadt |
bain-des-dames | 46.450507 | 6.858092 | La Tour-de-Peilz |
luscherz-two | 47.047519 | 7.152829 | Lüscherz |
impromptu_cudrefin | 46.964496 | 7.027936 | Cudrefin |
vira-spiaggia-left-of-river | 46.142900 | 8.838407 | Gambarogno |
linth_mollis | 47.131370 | 9.094768 | Glarus Nord |
seez | 47.119830 | 9.300251 | Walenstadt |
tolochenaz | 46.497509 | 6.482875 | Tolochenaz |
limmat_zuerich_suterdglauserp | 47.394539 | 8.513443 | Zürich |
aare_post | 47.116665 | 7.271953 | Port |
aare_bern_scheurerk | 46.970967 | 7.452586 | Bern |
strandbeiz | 47.215862 | 8.843098 | Rapperswil-Jona |
vierwaldstattersee_weggis_schoberls_1 | 47.044532 | 8.418569 | Weggis |
vierwaldstattersee_weggis_schoberls_2 | 47.043953 | 8.417308 | Weggis |
vierwaldstattersee_weggis_schoberls_3 | 47.029957 | 8.410801 | Weggis |
1.7.4. Inventar der Objekte#
Show code cell source
pd.set_option("display.max_rows", None)
complete_inventory = fdx.code_summary.copy()
complete_inventory.sort_values(by="quantity", ascending=False, inplace=True)
complete_inventory["quantity"] = complete_inventory["quantity"].map(lambda x: featuredata.thousandsSeparator(x, language))
complete_inventory["% of total"] = complete_inventory["% of total"].astype(int)
complete_inventory[unit_label] = complete_inventory[unit_label].astype(int)
complete_inventory.rename(columns=featuredata.inventory_table_de, inplace=True)
inventory_subsection = Paragraph("Inventar der Objekte", featuredata.subsection_title)
col_widths=[1.2*cm, 4.5*cm, 2.2*cm, 1.5*cm, 1.5*cm, 2.4*cm, 1.5*cm]
inventory_table = aStyledTableWithTitleRow(complete_inventory, title="Inventar der Objekte", colWidths=col_widths)
new_map_image = Image('resources/maps/survey_locations_all.jpeg', width=cm*16, height=12*cm, kind="proportional", hAlign= "CENTER")
map_caption = featuredata.defaultMapCaption(language="de")
new_components = [
KeepTogether([
featuredata.large_space,
location_subsection,
featuredata.small_space,
new_map_image,
Paragraph(map_caption, featuredata.caption_style),
featuredata.smaller_space,
pdf_table,
]),
featuredata.large_space,
inventory_table
]
pdfcomponents = featuredata.addToDoc(new_components, pdfcomponents)
complete_inventory
Objekte | Objekte (St.) | Anteil | p/100 m | Häufigkeitsrate | Material | |
---|---|---|---|---|---|---|
code | ||||||
G27 | Zigarettenfilter | 8 485 | 15 | 20 | 87 | Plastik |
Gfrags | Fragmentierte Kunststoffe | 7 400 | 13 | 18 | 86 | Plastik |
Gfoam | Expandiertes Polystyrol | 5 563 | 10 | 5 | 68 | Plastik |
G30 | Snack-Verpackungen | 3 325 | 6 | 9 | 85 | Plastik |
G67 | Industriefolie (Kunststoff) | 2 534 | 4 | 5 | 69 | Plastik |
G200 | Getränkeflaschen aus Glas, Glasfragmente | 2 136 | 3 | 3 | 65 | Glas |
G112 | Industriepellets (Nurdles) | 1 968 | 3 | 0 | 30 | Plastik |
G74 | Schaumstoffverpackungen/Isolierung | 1 702 | 3 | 1 | 53 | Plastik |
G95 | Wattestäbchen/Tupfer | 1 406 | 2 | 1 | 50 | Plastik |
G117 | Styropor < 5mm | 1 209 | 2 | 0 | 25 | Plastik |
G89 | Kunststoff-Bauabfälle | 992 | 1 | 1 | 52 | Plastik |
G941 | Verpackungsfolien, nicht für Lebensmittel | 894 | 1 | 0 | 38 | Plastik |
G178 | Kronkorken, Lasche von Dose/Ausfreisslachen | 700 | 1 | 1 | 52 | Metall |
G25 | Tabak; Kunststoffverpackungen, Behälter | 649 | 1 | 0 | 46 | Plastik |
G21 | Getränke-Deckel, Getränkeverschluss | 623 | 1 | 0 | 37 | Plastik |
G106 | Kunststofffragmente eckig <5mm | 592 | 1 | 0 | 20 | Plastik |
G98 | Windeln – Feuchttücher | 588 | 1 | 0 | 26 | Plastik |
G24 | Ringe von Plastikflaschen/Behältern | 541 | 0 | 0 | 42 | Plastik |
G177 | Verpackungen aus Aluminiumfolie | 521 | 0 | 0 | 47 | Metall |
G10 | Lebensmittelbehälter zum einmaligen Gebrauch a... | 448 | 0 | 0 | 31 | Plastik |
G23 | unidentifizierte Deckel | 423 | 0 | 0 | 35 | Plastik |
G156 | Papierfragmente | 420 | 0 | 0 | 32 | Papier |
G35 | Strohhalme und Rührstäbchen | 419 | 0 | 0 | 42 | Plastik |
G70 | Schrotflintenpatronen | 391 | 0 | 0 | 21 | Plastik |
G31 | Schleckstengel, Stengel von Lutscher | 381 | 0 | 0 | 37 | Plastik |
G73 | Gegenstände aus Schaumstoff & Teilstücke (nich... | 335 | 0 | 0 | 23 | Plastik |
G33 | Einwegartikel; Tassen/Becher & Deckel | 328 | 0 | 0 | 34 | Plastik |
G32 | Spielzeug und Partyartikel | 320 | 0 | 0 | 38 | Plastik |
G904 | Feuerwerkskörper; Raketenkappen | 301 | 0 | 0 | 28 | Plastik |
G3 | Einkaufstaschen, Shoppingtaschen | 285 | 0 | 0 | 18 | Plastik |
G22 | Deckel für Chemikalien, Reinigungsmittel (Ohne... | 257 | 0 | 0 | 19 | Plastik |
G211 | Sonstiges medizinisches Material | 256 | 0 | 0 | 31 | Plastik |
G922 | Etiketten, Strichcodes | 252 | 0 | 0 | 27 | Plastik |
G66 | Umreifungsbänder; Hartplastik für Verpackung f... | 248 | 0 | 0 | 32 | Plastik |
G100 | Medizin; Behälter/Röhrchen/Verpackungen | 244 | 0 | 0 | 28 | Plastik |
G923 | Taschentücher, Toilettenpapier, Servietten, Pa... | 241 | 0 | 0 | 25 | Papier |
G103 | Kunststofffragmente rund <5mm | 238 | 0 | 0 | 3 | Plastik |
G921 | Keramikfliesen und Bruchstücke | 231 | 0 | 0 | 13 | Glas |
G96 | Hygienebinden/Höscheneinlagen/Tampons und Appl... | 215 | 0 | 0 | 18 | Plastik |
G50 | Schnur < 1cm | 205 | 0 | 0 | 29 | Plastik |
G153 | Papierbecher, Lebensmittelverpackungen aus Pap... | 201 | 0 | 0 | 19 | Papier |
G125 | Luftballons und Luftballonstäbchen | 170 | 0 | 0 | 20 | Gummi |
G91 | Bio-Filtermaterial / Trägermaterial aus Kunst... | 162 | 0 | 0 | 19 | Plastik |
G159 | Kork | 162 | 0 | 0 | 21 | Holz |
G38 | Abdeckungen; Kunststoffverpackungen, Folien zu... | 160 | 0 | 0 | 4 | Plastik |
G940 | Schaumstoff EVA (flexibler Kunststoff) | 158 | 0 | 0 | 7 | Plastik |
G208 | Glas oder Keramikfragmente >2.5 cm | 145 | 0 | 0 | 10 | Glas |
G186 | Industrieschrott | 141 | 0 | 0 | 15 | Metall |
G213 | Paraffinwachs | 138 | 0 | 0 | 13 | Chemikalien |
G165 | Glacestengel (Eisstiele), Zahnstocher, Essstäb... | 126 | 0 | 0 | 15 | Holz |
G204 | Baumaterial; Ziegel, Rohre, Zement | 125 | 0 | 0 | 9 | Glas |
G155 | Feuerwerkspapierhülsen und -fragmente | 124 | 0 | 0 | 6 | Papier |
G93 | Kabelbinder | 121 | 0 | 0 | 16 | Plastik |
G149 | Papierverpackungen | 121 | 0 | 0 | 8 | Papier |
G152 | Papier &Karton;Zigarettenschachteln, Papier/Ka... | 120 | 0 | 0 | 13 | Papier |
G90 | Blumentöpfe aus Plastik | 119 | 0 | 0 | 14 | Plastik |
G137 | Kleidung, Handtücher und Lappen | 118 | 0 | 0 | 13 | Stoff |
G908 | Klebeband; elektrisch, isolierend | 117 | 0 | 0 | 15 | Plastik |
G203 | Geschirr aus Keramik oder Glas, Tassen, Teller... | 115 | 0 | 0 | 11 | Glas |
G936 | Folien für Gewächshäuser | 110 | 0 | 0 | 12 | Plastik |
G34 | Besteck, Teller und Tabletts | 109 | 0 | 0 | 16 | Plastik |
G905 | Haarspangen, Haargummis, persönliche Accessoir... | 105 | 0 | 0 | 18 | Plastik |
G198 | Andere Metallteile < 50 cm | 104 | 0 | 0 | 16 | Metall |
G914 | Büroklammern, Wäscheklammern, Gebrauchsgegenst... | 99 | 0 | 0 | 12 | Plastik |
G131 | Gummibänder | 94 | 0 | 0 | 16 | Gummi |
G191 | Draht und Gitter | 90 | 0 | 0 | 12 | Metall |
G28 | Stifte, Deckel, Druckbleistifte usw. | 88 | 0 | 0 | 13 | Plastik |
G115 | Schaumstoff <5mm | 88 | 0 | 0 | 4 | Plastik |
G124 | Andere Kunststoff | 87 | 0 | 0 | 8 | Plastik |
G87 | Abdeckklebeband/Verpackungsklebeband | 87 | 0 | 0 | 14 | Plastik |
G148 | Kartonkisten und Stücke | 85 | 0 | 0 | 8 | Papier |
G928 | Bänder und Schleifen | 84 | 0 | 0 | 8 | Plastik |
G26 | Feuerzeug | 83 | 0 | 0 | 14 | Plastik |
G146 | Papier, Karton | 82 | 0 | 0 | 6 | Papier |
G175 | Getränkedosen (Dosen, Getränke) | 76 | 0 | 0 | 11 | Metall |
G157 | Papier | 66 | 0 | 0 | 6 | Papier |
G7 | Getränkeflaschen < = 0,5 l | 65 | 0 | 0 | 9 | Plastik |
G4 | Kleine Plastikbeutel; Gefrierbeutel, Zippsäckc... | 64 | 0 | 0 | 8 | Plastik |
G142 | Seil, Schnur oder Netze | 62 | 0 | 0 | 10 | Stoff |
G135 | Kleidung, Fussbekleidung, Kopfbedeckung, Hands... | 61 | 0 | 0 | 9 | Stoff |
G105 | Kunststofffragmente subangulär <5mm | 59 | 0 | 0 | 2 | Plastik |
G927 | Plastikschnur von Rasentrimmern, die zum Schne... | 57 | 0 | 0 | 8 | Plastik |
G201 | Gläser, einschliesslich Stücke | 55 | 0 | 0 | 7 | Glas |
G942 | Kunststoffspäne von Drehbänken, CNC-Bearbeitung | 53 | 0 | 0 | 8 | Plastik |
G194 | Kabel, Metalldraht oft in Gummi- oder Kunststo... | 52 | 0 | 0 | 10 | Metall |
G939 | Kunststoffblumen, Kunststoffpflanzen | 49 | 0 | 0 | 6 | Plastik |
G20 | Laschen und Deckel | 48 | 0 | 0 | 6 | Plastik |
G65 | Eimer | 48 | 0 | 0 | 6 | Plastik |
G943 | Zäune Landwirtschaft, Kunststoff | 47 | 0 | 0 | 5 | Plastik |
G144 | Tampons | 46 | 0 | 0 | 3 | Stoff |
G48 | Seile, synthetische | 46 | 0 | 0 | 8 | Plastik |
G134 | Sonstiges Gummi | 45 | 0 | 0 | 8 | Gummi |
G210 | Sonstiges Glas/Keramik Materialien | 43 | 0 | 0 | 3 | Glas |
G170 | Holz (verarbeitet) | 43 | 0 | 0 | 6 | Holz |
G937 | Pheromonköder für Weinberge | 38 | 0 | 0 | 4 | Plastik |
G101 | Robidog Hundekot-Säcklein, andere Hundekotsäck... | 37 | 0 | 0 | 8 | Plastik |
G917 | Blähton | 36 | 0 | 0 | 4 | Glas |
G59 | Monofile Angelschnur (Angeln) | 35 | 0 | 0 | 6 | Plastik |
G944 | Pelletmasse aus dem Spritzgussverfahren | 34 | 0 | 0 | 0 | Plastik |
G2 | Säcke, Taschen | 32 | 0 | 0 | 4 | Plastik |
G12 | Kosmetika, Behälter für Körperpflegeprodukte, ... | 31 | 0 | 0 | 5 | Plastik |
G126 | Bälle | 30 | 0 | 0 | 5 | Gummi |
G207 | Tonkrüge zum Fangen von Kraken | 30 | 0 | 0 | 0 | Glas |
G901 | Medizinische Masken, synthetische | 29 | 0 | 0 | 6 | Plastik |
G931 | (Absperr)band für Absperrungen, Polizei, Baust... | 28 | 0 | 0 | 3 | Plastik |
G99 | Spritzen - Nadeln | 28 | 0 | 0 | 6 | Plastik |
G918 | Sicherheitsnadeln, Büroklammern, kleine Gebrau... | 28 | 0 | 0 | 5 | Metall |
G158 | Sonstige Gegenstände aus Papier | 27 | 0 | 0 | 2 | Papier |
G919 | Nägel, Schrauben, Bolzen usw. | 27 | 0 | 0 | 3 | Metall |
G167 | Streichhölzer oder Feuerwerke | 26 | 0 | 0 | 2 | Holz |
G933 | Taschen, Etuis für Zubehör, Brillen, Elektroni... | 26 | 0 | 0 | 4 | Plastik |
G154 | Zeitungen oder Zeitschriften | 25 | 0 | 0 | 2 | Papier |
G6 | Flaschen und Behälter aus Kunststoff, nicht fü... | 25 | 0 | 0 | 2 | Plastik |
G161 | Verarbeitetes Holz | 24 | 0 | 0 | 4 | Holz |
G182 | Angeln; Haken, Gewichte, Köder, Senkblei, usw. | 23 | 0 | 0 | 3 | Metall |
G8 | Getränkeflaschen > 0,5L | 23 | 0 | 0 | 3 | Plastik |
G114 | Folien <5mm | 22 | 0 | 0 | 1 | Plastik |
G145 | Andere Textilien | 22 | 0 | 0 | 4 | Stoff |
G43 | Sicherheitsetiketten, Siegel für Fischerei ode... | 21 | 0 | 0 | 2 | Plastik |
G68 | Fiberglas-Fragmente | 20 | 0 | 0 | 4 | Plastik |
G133 | Kondome einschließlich Verpackungen | 20 | 0 | 0 | 4 | Gummi |
G929 | Elektronik und Teile; Sensoren, Headsets usw. | 20 | 0 | 0 | 4 | Metall |
G930 | Schaumstoff-Ohrstöpsel | 20 | 0 | 0 | 4 | Plastik |
G925 | Pakete: Trockenmittel/Feuchtigkeitsabsorber, m... | 19 | 0 | 0 | 4 | Plastik |
G176 | Konservendosen (Lebensmitteldosen) | 19 | 0 | 0 | 3 | Metall |
G938 | Zahnstocher, Zahnseide Kunststoff | 18 | 0 | 0 | 4 | Plastik |
G926 | Kaugummi, enthält oft Kunststoffe | 18 | 0 | 0 | 3 | Plastik |
G36 | Säcke aus strapazierfähigem Kunststoff für 25 ... | 18 | 0 | 0 | 2 | Plastik |
G118 | Kleine Industrielle Kügelchen <5mm | 17 | 0 | 0 | 2 | Plastik |
G11 | Kosmetika für den Strand, z.B. Sonnencreme | 17 | 0 | 0 | 3 | Plastik |
G123 | Polyurethan-Granulat < 5mm | 17 | 0 | 0 | 0 | Plastik |
G64 | Kotflügel | 16 | 0 | 0 | 1 | Plastik |
G119 | Folienartiger Kunststoff (>1mm) | 16 | 0 | 0 | 1 | Plastik |
G49 | Seile > 1cm | 14 | 0 | 0 | 2 | Plastik |
G113 | Fäden <5mm | 14 | 0 | 0 | 1 | Plastik |
G199 | Andere Metallteile > 50 cm | 13 | 0 | 0 | 1 | Metall |
G197 | sonstiges Metall | 13 | 0 | 0 | 3 | Metall |
G29 | Kämme, Bürsten und Sonnenbrillen | 13 | 0 | 0 | 3 | Plastik |
G195 | Batterien (Haushalt) | 12 | 0 | 0 | 2 | Metall |
G128 | Reifen und Antriebsriemen | 12 | 0 | 0 | 2 | Gummi |
G916 | Bleistifte und Bruchstücke | 12 | 0 | 0 | 2 | Holz |
G136 | Schuhe | 11 | 0 | 0 | 2 | Stoff |
G37 | Netzbeutel, Netztasche, Netzsäcke | 11 | 0 | 0 | 2 | Plastik |
G900 | Handschuhe Latex, persönliche Schutzausrüstung | 10 | 0 | 0 | 2 | Gummi |
G906 | Kaffeekapseln Aluminium | 10 | 0 | 0 | 1 | Metall |
G61 | Sonstiges Angelzubehör | 9 | 0 | 0 | 2 | Plastik |
G913 | Schnuller | 8 | 0 | 0 | 2 | Plastik |
G129 | Schläuche und Gummiplatten | 7 | 0 | 0 | 1 | Gummi |
G104 | Kunststofffragmente abgerundet / rundlich <5m... | 7 | 0 | 0 | 1 | Plastik |
G17 | Kartuschen von Kartuschen-spritzpistolen | 7 | 0 | 0 | 1 | Plastik |
G122 | Kunststofffragmente (>1mm) | 7 | 0 | 0 | 0 | Plastik |
G19 | Autoteile | 7 | 0 | 0 | 1 | Plastik |
G40 | Handschuhe Haushalt/Garten | 7 | 0 | 0 | 1 | Plastik |
G147 | Papiertragetaschen, (Papiertüten) | 6 | 0 | 0 | 1 | Papier |
G151 | Tetrapack, Kartons | 6 | 0 | 0 | 1 | Papier |
G915 | Reflektoren, Mobilitätsartikel aus Kunststoff | 6 | 0 | 0 | 1 | Plastik |
G181 | Geschirr aus Metall, Tassen, Besteck usw. | 6 | 0 | 0 | 1 | Metall |
G97 | Behälter von Toilettenerfrischer | 6 | 0 | 0 | 1 | Plastik |
G141 | Teppiche | 6 | 0 | 0 | 1 | Stoff |
G171 | Sonstiges Holz < 50cm | 6 | 0 | 0 | 1 | Holz |
G5 | Plastiksäcke/ Plastiktüten | 6 | 0 | 0 | 1 | Plastik |
G107 | Zylindrische Pellets <5mm | 6 | 0 | 0 | 1 | Plastik |
G71 | Schuhe Sandalen | 5 | 0 | 0 | 1 | Plastik |
G138 | Schuhe und Sandalen | 5 | 0 | 0 | 1 | Stoff |
G108 | Scheibenförmige Pellets <5mm | 5 | 0 | 0 | 1 | Plastik |
G92 | Köderbehälter | 5 | 0 | 0 | 1 | Plastik |
G903 | Behälter und Packungen für Handdesinfektionsmi... | 5 | 0 | 0 | 1 | Plastik |
G172 | Sonstiges Holz > 50 cm | 4 | 0 | 0 | 1 | Holz |
G907 | Kaffeekapseln aus Kunststoff | 4 | 0 | 0 | 1 | Plastik |
G13 | Flaschen, Behälter, Fässer zum Transportieren ... | 4 | 0 | 0 | 0 | Plastik |
G39 | Handschuhe | 4 | 0 | 0 | 0 | Plastik |
G62 | Schwimmer für Netze | 4 | 0 | 0 | 0 | Plastik |
G53 | Netze und Teilstücke < 50cm | 4 | 0 | 0 | 0 | Plastik |
G52 | Netze und Teilstücke | 4 | 0 | 0 | 1 | Plastik |
G111 | Kugelförmige Pellets < 5mm | 4 | 0 | 0 | 1 | Plastik |
G945 | Rasierklingen | 4 | 0 | 0 | 1 | Metall |
G934 | Sandsäcke, Kunststoff für Hochwasser- und Eros... | 3 | 0 | 0 | 0 | Plastik |
G116 | Granulat <5mm | 3 | 0 | 0 | 0 | Plastik |
G109 | Flache Pellets <5mm | 3 | 0 | 0 | 0 | Plastik |
G102 | Flip-Flops | 3 | 0 | 0 | 0 | Plastik |
G174 | Aerosolspraydosen | 3 | 0 | 0 | 0 | Metall |
G902 | Medizinische Masken, Stoff | 3 | 0 | 0 | 0 | Stoff |
G63 | Bojen | 3 | 0 | 0 | 0 | Plastik |
G179 | Einweg Grill | 3 | 0 | 0 | 0 | Metall |
G202 | Glühbirnen | 3 | 0 | 0 | 0 | Glas |
G150 | Milchkartons, Tetrapack | 3 | 0 | 0 | 0 | Papier |
G143 | Segel und Segeltuch | 3 | 0 | 0 | 0 | Stoff |
G140 | Sack oder Beutel (Tragetasche), Jute oder Hanf | 3 | 0 | 0 | 0 | Stoff |
G139 | Rucksäcke | 3 | 0 | 0 | 0 | Stoff |
G41 | Handschuhe Industriell/Professionell | 3 | 0 | 0 | 0 | Plastik |
G14 | Flaschen für Motoröl (Motorölflaschen) | 3 | 0 | 0 | 0 | Plastik |
G935 | Gummi-Puffer für Geh- und Wanderstöcke und Tei... | 2 | 0 | 0 | 0 | Plastik |
G173 | Sonstiges | 2 | 0 | 0 | 0 | Holz |
G999 | Keine Gegenstände bei dieser Erhebung/Studie g... | 2 | 0 | 0 | 0 | Unbekannt |
G214 | Öl/Teer | 2 | 0 | 0 | 0 | Chemikalien |
G185 | Behälter mittlerer Grösse | 2 | 0 | 0 | 0 | Metall |
G132 | Schwimmer (Angeln) | 2 | 0 | 0 | 0 | Gummi |
G188 | Andere Kanister/Behälter < 4 L | 2 | 0 | 0 | 0 | Metall |
G55 | Angelschnur (verwickelt) | 2 | 0 | 0 | 0 | Plastik |
G932 | Bio-Beads, Mikroplastik für die Abwasserbehand... | 2 | 0 | 0 | 0 | Plastik |
G190 | Farbtöpfe, Farbbüchsen, ( Farbeimer) | 2 | 0 | 0 | 0 | Metall |
G9 | Flaschen und Behälter für Reinigungsmittel und... | 1 | 0 | 0 | 0 | Plastik |
G166 | Farbpinsel | 1 | 0 | 0 | 0 | Holz |
G84 | CD oder CD-Hülle | 1 | 0 | 0 | 0 | Plastik |
G196 | Grosse metallische Gegenstände | 1 | 0 | 0 | 0 | Metall |
G51 | Fischernetz | 1 | 0 | 0 | 0 | Plastik |
G193 | Teile von Autos und Autobatterien | 1 | 0 | 0 | 0 | Metall |
G60 | Lichtstab, Knicklicht, Glow-sticks | 1 | 0 | 0 | 0 | Plastik |
G183 | Teile von Angelhaken | 1 | 0 | 0 | 0 | Metall |
G94 | Tischtuch | 1 | 0 | 0 | 0 | Plastik |
G205 | Leuchtstoffröhren | 0 | 0 | 0 | 0 | Glas |
G168 | Holzbretter | 0 | 0 | 0 | 0 | Holz |
G180 | Haushaltsgeräte | 0 | 0 | 0 | 0 | Metall |
G88 | Telefon inkl. Teile | 0 | 0 | 0 | 0 | Plastik |
G708 | Skistöcke | 0 | 0 | 0 | 0 | Metall |
G162 | Kisten | 0 | 0 | 0 | 0 | Holz |
G160 | Paletten | 0 | 0 | 0 | 0 | Holz |
G707 | Skiausrüstungsetikett | 0 | 0 | 0 | 0 | Plastik |
G713 | Skiförderband | 0 | 0 | 0 | 0 | Plastik |
G712 | Skihandschuhe | 0 | 0 | 0 | 0 | Stoff |
G711 | Handwärmer | 0 | 0 | 0 | 0 | Plastik |
G710 | Ski / Snowboards (Ski, Befestigungen und ander... | 0 | 0 | 0 | 0 | Plastik |
G56 | Verhedderte Netze | 0 | 0 | 0 | 0 | Plastik |
G709 | Teller von Skistock (runder Teil aus Plastik u... | 0 | 0 | 0 | 0 | Plastik |
G702 | Pistenmarkierungspfosten (Holz) | 0 | 0 | 0 | 0 | Holz |
G703 | Pistenmarkierungspfosten (Plastik) | 0 | 0 | 0 | 0 | Plastik |
G704 | Seilbahnbürsten | 0 | 0 | 0 | 0 | Plastik |
G705 | Schrauben und Bolzen | 0 | 0 | 0 | 0 | Metall |
G706 | Skiabonnement | 0 | 0 | 0 | 0 | Plastik |
G1 | Sixpack-Ringe | 0 | 0 | 0 | 0 | Plastik |